Let's learn how to create a Grid with the new native Grid in Kendo UI for Vue. The new native Grid is built with Vue from the ground up.
In the first post in this two part series, we will learn how to create a Kendo UI native Grid for Vue. The new native Grid is built with Vue from the ground up. One way the native grid differs from the grid wrapper is that the native grid doesn’t depend on jQuery. The other difference is that the DataSource
component is no longer required to load data. The data can be defined directly inside the component. Coming up, we will see how to create a native grid using local and remote data. Then we will add a pager to the grid to split the data into pages.
First, we will initialize our project using the Vue webpack-simple template. Inside the project directory, we will install a theme, the grid, the globalization package, and the vue-class-component using the following commands:
npm install --save @progress/kendo-theme-default
npm install --save @progress/kendo-vue-grid
npm install --save @progress/kendo-vue-intl
npm install --save vue-class-component
Next, we import the theme and the grid in our main.js
file and register the grid component globally.
import Vue from 'vue'
import App from './App.vue'
import '@progress/kendo-theme-default/dist/all.css'
import { Grid } from '@progress/kendo-vue-grid'
Vue.component('Grid', Grid);
new Vue({
el: '#app',
render: h => h(App)
})
In this first example, we will define our data locally. The data is provided by uinames.com. We will add our grid component to the template in the App.vue
file and set the data-items
and columns
property. The data-items
property sets the grid’s data. The columns
property sets the fields of the grid. This is the updated App.vue
file:
<template>
<div id="app">
<Grid :style="{height: '200px'}"
:data-items="dataItems"
:columns="columns">
</Grid>
</div>
</template>
<script>
export default {
name: 'app',
data () {
return {
dataItems: [
{
"name": "Ruxanda",
"surname": "Corbea",
"gender": "female",
"region": "Romania"
},
{
"name": "Paula",
"surname": "Acevedo",
"gender": "female",
"region": "Mexico"
},
{
"name": "David",
"surname": "Dediu",
"gender": "male",
"region": "Romania"
},
{
"name": "Urmila",
"surname": "Belbase",
"gender": "female",
"region": "Nepal"
}],
columns: [
{ field: 'name'},
{ field: 'surname'},
{ field: 'gender'},
{ field: 'region' }
],
};
}
}
</script>
<style>
#app {
font-family: 'Avenir', Helvetica, Arial, sans-serif;
color: #2c3e50;
margin-top: 60px;
}
</style>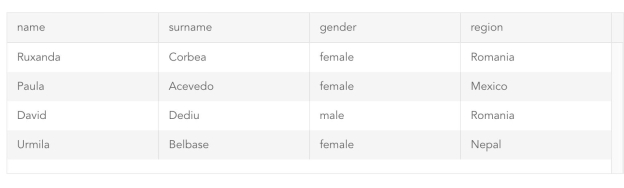
Using the same data, we will build a grid from a remote data source. We will start with an empty dataItems
array. This time we will use axios to fetch the data from an API. Then we will fill the dataItems
array with the result. The API call will take place within the mounted
lifecycle event. First, we will install axios with the following command:
npm install --save axios
Then we import axios in the script of our App.vue
file and load the data from within the mounted
lifecycle event. This is the updated script:
<script>
import axios from 'axios'
export default {
name: 'app',
data () {
return {
dataItems: [],
columns: [
{ field: 'name'},
{ field: 'surname'},
{ field: 'gender'},
{ field: 'region' }
],
}
},
mounted () {
axios
.get('https://uinames.com/api/?amount=25')
.then(response => {
this.dataItems = response.data
})
}
}
</script>
By default, all of the records in the grid show on one page. To implement paging, we need to configure several properties. We must set the pageable
, skip
, take
, and total
properties of our grid as well as the pagechange
event. The pageable
property configures the pager for the grid. The skip
property is the number of records the pager will skip. The take
property is the number of records to show for each page. The total
is the total number of data items. And pagechange
is the event that fires when the page is changed. This is the updated template:
<template>
<div id="app">
<Grid :style="{height: '200px'}"
:data-items="result"
:columns="columns"
:pageable="true"
:skip="skip"
:take="take"
:total="total"
@pagechange="pageChange">
</Grid>
</div>
</template>
The data-items
property should be set to the data for the current page. To achieve this, we create a computed method named result
that calculates which records to take from the list of data items. The skip
property is initialized to 0 and take
is initialized to 10. We create a computed method for total
that returns the number of data items. Last, we add a pageChange
method which updates the skip
and take
properties. This is the updated script:
<script>
import axios from 'axios'
export default {
name: 'app',
data () {
return {
dataItems: [],
columns: [
{ field: 'name'},
{ field: 'surname'},
{ field: 'gender'},
{ field: 'region' }
],
skip: 0,
take: 10,
}
},
computed: {
result () {
return this.dataItems.slice(this.skip, this.take + this.skip)
},
total () {
return this.dataItems.length
}
},
mounted () {
axios
.get('https://uinames.com/api/?amount=25')
.then(response => {
this.dataItems = response.data
})
},
methods: {
pageChange(event) {
this.skip = event.page.skip;
this.take = event.page.take;
}
}
}
</script>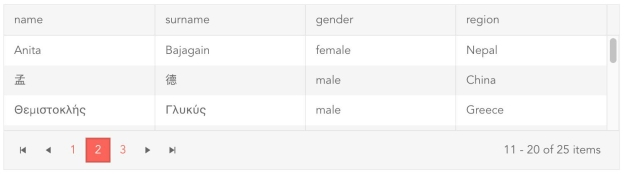
See the final project repo here: https://github.com/albertaw/kendoui-vue-native-grid
First, we saw how to create a grid using local data. That required setting the data-items
property equal to a list defined in the data and setting the columns
property. Then we created the grid from a remote data source by using axios to make an API call within Vue’s mounted
lifecycle event. Last, we added paging to the grid. We had to set the pageable
, skip
, take
, and total
properties and handle the pagechange
event.
In the next post, we will see how to edit records in the grid.
Want to start taking advantage of the more than 70+ ready-made Kendo UI components, like the Grid or Scheduler? You can begin a free trial of Kendo UI today and start developing your apps faster.
Looking for UI component to support specific frameworks? Check out Kendo UI for Angular, KendoReact, or Kendo UI for jQuery.
Alberta is a software developer and writer from New Orleans. Learn more about Alberta at github.com/albertaw.