I have a problem in the Grid, I have created a calculated field that for the example is the calculated date, this field does not belong to the TelerikGrid Data model, when clicking on the column header of said field an error occurs. It is very necessary to perform calculations and display them in a column, the data in my project is generated from a dbcontext, so I do not want to alter the SQL tables that I have been managing. There is a way to avoid this error, the error occurs even when removing the filter from the column, please, if someone has a solution it would help me out of trouble.
https://blazorrepl.telerik.com/GRPYFhvp14M25Tft34
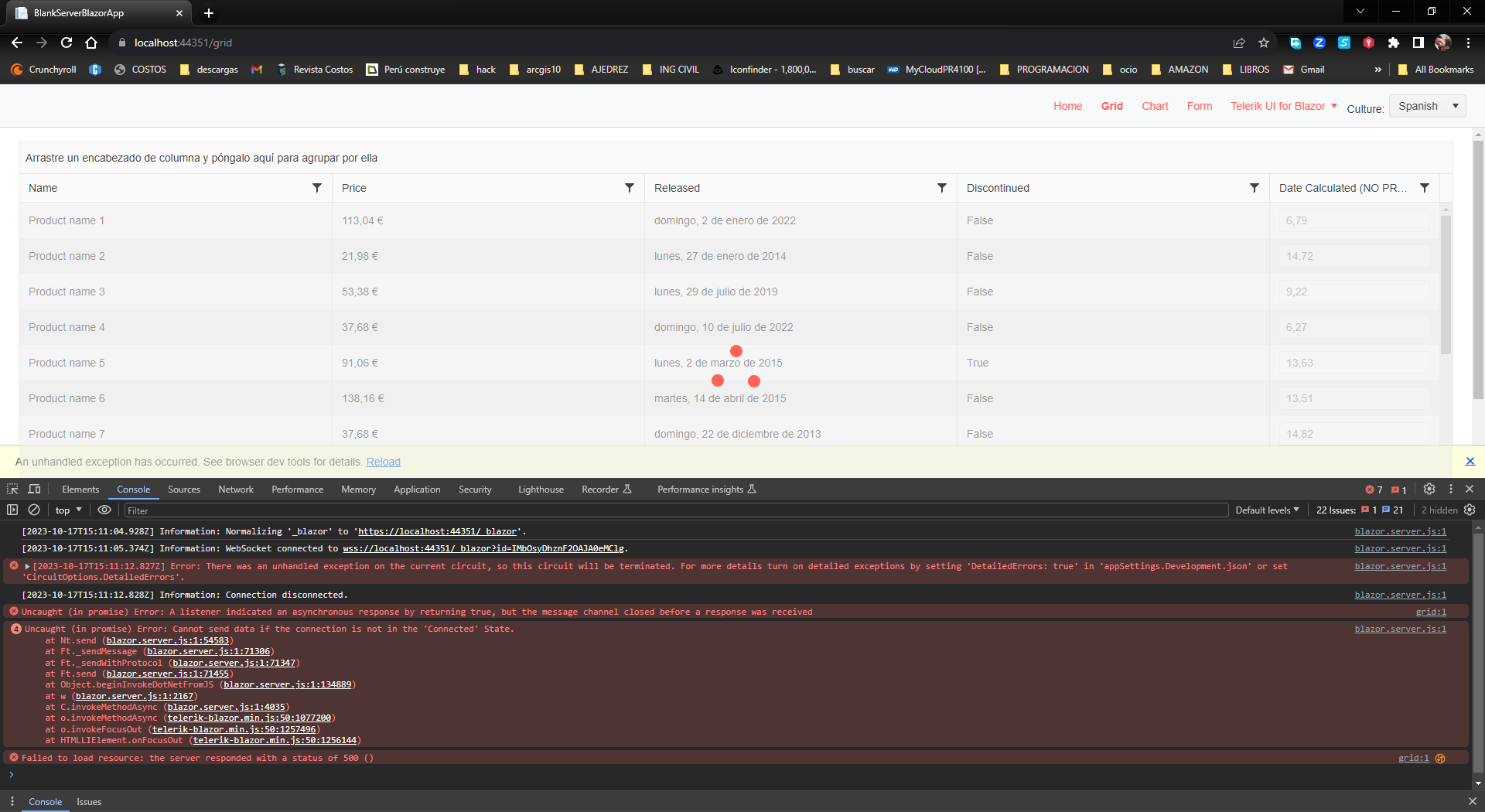
Kind regards.
https://blazorrepl.telerik.com/GRPYFhvp14M25Tft34
@page "/Grid"
@using Telerik.Blazor.Services
@using Telerik.FontIcons;
@using Telerik.Blazor.Components.Grid
<TelerikGrid Data="@GridData" Height="550px" FilterMode="@GridFilterMode.FilterMenu"
Sortable="true" Pageable="true" PageSize="20" Groupable="true" Resizable="true" Reorderable="true">
<GridColumns>
<GridColumn Field="@nameof(Product.Name)" Title="Name"/>
<GridColumn Field="@nameof(Product.Price)" DisplayFormat="{0:C2}" />
<GridColumn Field="@nameof(Product.Released)" DisplayFormat="{0:D}" />
<GridColumn Field="@nameof(Product.Discontinued)" />
<GridColumn Field="Date Calculated (NO PRESENT IN MODEL)" Width="220px" DisplayFormat="{0:dddd, dd MMM yyyy}">
<Template>
@{
var fecha = (Product)context;
double FechaCalculada;
TimeSpan diferencia = DateTime.Today - fecha.Released;
double difDouble = 5+diferencia.TotalDays / 365.25; // considerando años bisiestos
FechaCalculada = Math.Round(difDouble, 2);
}
<TelerikNumericTextBox Decimals="2" @bind-Value=@FechaCalculada DebounceDelay="200" Enabled=false Arrows="false" />
</Template>
</GridColumn>
</GridColumns>
</TelerikGrid>
@code {
private List<Product> GridData { get; set; }
protected override void OnInitialized()
{
GridData = new List<Product>();
var rnd = new Random();
for (int i = 1; i <= 30; i++)
{
GridData.Add(new Product
{
Id = i,
Name = "Product name " + i,
Price = (decimal)(rnd.Next(1, 50) * 3.14),
Released = DateTime.Now.AddDays(-rnd.Next(1, 365)).AddYears(-rnd.Next(1, 10)).Date,
Discontinued = i % 5 == 0
});
}
}
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
public DateTime Released { get; set; }
public bool Discontinued { get; set; }
}
}
Kind regards.