Updated April 2022 to include links to more information.
Understanding Angular’s data binding types is important when building Angular applications. Learn about property binding and interpolation so you can understand which method best suits the task at hand.
When building applications using Angular, you come in contact with a couple of ways for displaying data on the view. Property binding and interpolation are the data binding types in Angular, used for moving data from the component to the template.
Data binding is a very important and powerful aspect of software development. It involves the concept of defining the communication between a component and its respective views. Data binding allows for dynamism and interactivity in applications.
There are about four types of data binding available in Angular:
// component.html
<p>My name is {{name}}</p>
<button (click)="updateName()">Update button</button>
// component.ts
@Component({
templateUrl: 'component.html',
selector: 'app-component',
})
export class Component {
name = 'Peter';
updateName() {
this.name = 'John';
}
}
//component.html
<p>{{ name }}</p>
// component.ts
@Component({
templateUrl: 'component.html',
selector: 'app-component',
})
export class Component {
name = 'Peter';
}
[]
syntax for data binding. An example is setting the disabled
state of a button.
// component.html
<button [disabled]="buttonDisabled"></button>
// component.ts
@Component({
templateUrl: 'component.html',
selector: 'app-component',
})
export class Component {
buttonDisabled = true;
}
Interpolation, like we mentioned above, is a mechanism that allows the integration of defined string values into text within HTML tags and attribute assignments in the presentation layer (view). Interpolation makes use of the {{ }}
double
curly braces syntax to embed template expressions that will be converted by Angular into marked-up text in the view layer.
<p> Score count: {{5 + 5}} </p>
The snippet above shows an example of string interpolation. In the example above, Angular will run the expression between the curly braces and will render 10
as the element’s text rather than 5 + 5
. Not all expressions
run between the braces are allowed. You can’t declare a variable:
<p> {{ const score = 5 + 5 </p>
The above example is not allowed and will throw an error. Another example of expressions not allowed is initializing a class:
<p> {{ new Date() }} </p>
This will throw an error, as declaring objects when using string interpolation isn’t allowed. Functions with a return value on the other hand can be called, and Angular will evaluate the expression and convert it to a string.
<p> {{ convertToDate() }} </p>
The convertToDate
function might be something similar to:
function convertToDate(){
const date = new Date();
return date.toLocaleDateString();
}
Basically, you cannot use JavaScript expressions that have side effects like:
=, +=, -=, …
)new
, instanceOf
, typeOf
, for
, while
, etc.;
or ,
++
and --
;Recent ES6 operators are also exempted from interpolation, same with bitwise operators like |
and &
.
Template expressions are most often used when using interpolation in Angular. Template expression typically produces a value within the double curly braces that Angular executes and binds to the property of a target being an HTML element, component or directive.
The context of an expression is typically that of a component instance, but an expression can also refer to the properties of view model, like a form element for instance.
<p> {{beverageInput.value}} </p>
<select #beverageInput>
<option value="milo">Milo</option>
<option value="coke">Coke</option>
<option value="fanta">Fanta</option>
</select>
In the snippet above, we declared a template reference variable. A template reference variable is a value used to attach the reference of an element to a variable. In normal JavaScript, it is similar to doing this:
const beverageInput = document.querySelector('select');
But with a template variable, you can easily achieve this by attaching a value to the element starting with a pound/hash symbol #
.
Going back to the example above of declaring a template variable, we declared a variable called beverageInput
and tried to get the value of the variable. Angular sees this and immediately gets the value of the element attached to the variable
and displays it. Even if the value of the element is updated, the text between the p
element isn’t updated. This current implementation is static; to make it dynamic, we’ll update the element to use an event binding input
:
<select #beverageInput (input)="0">
<option value="milo">Milo</option>
<option value="coke">Coke</option>
<option value="fanta">Fanta</option>
</select>
Now when the value of the element is updated, the interpolated value is also updated.
When working with string interpolation, it is important to know the guidelines and limitations of template expressions:
Property binding is the base method of binding in Angular, it involves binding values to DOM properties of HTML elements. It is a one-way binding method, as values go from the component to the template layer and changes made in the component updates the properties bound in the template.
Properties bound to an element are always presented using square brackets []
. The values shouldn’t be confused with HTML attributes of the elements. Elements are typically represented as JavaScript DOM objects and their attributes
are represented as a properties of the DOM.
In Angular applications, we can attach properties to DOM using values declared in the component. Using the square brackets syntax, we can bind properties to DOM elements; the property to be bound can also be prefixed with bind-
. We can
bind to the DOM using these two methods:
//component.html
<img [alt]="animal.name" [src]="animal.image" />
<img bind-alt="animal.name" bind-src="animal.image"
@Component({
selector: 'app-component',
templateUrl: 'component.html'
})
export class AppComponent{
animal = {
name: 'Lion',
image: './assets/images/lion.jpg'
}
}
The above snippet binds data to the alt
and src
properties of the img
element. Once the page rendering is complete, the browser will display the image and the resulting alt
attribute.
After execution, both methods will be evaluated and the values of the animal object will be bound to the img
tag. Both the approaches produce the same result. The first one uses the square brackets syntax, second a bind-
prefix. There is no difference in the way they are executed on a page.
To render HTML using property binding, we can use the innerHTML
property. This property takes the assigned value of the attribute and displays it as the text content of the tag. You can define a string variable containing HTML elements
in the component end and display it using the method similar to the example below:
// component.html
<p [innerHTML]="nameDetails"></p>
// component.ts
@Component({
selector: 'app-component',
templateUrl: 'component.html'
})
export class AppComponent {
nameDetails = 'The name is Bond <b>James Bond<b/>';
}
When rendered, Angular parses the b
tag, and the text rendered within it is bold rather than rendering it as a normal string. When using the innerHTML
attribute, all HTML tags are allowed with exception of the script
tag. If the script tag was set to the nameDetails
value, it would render the element’s text content as a string rather than parsing it as an element. If the example below is attempted, the expression within the <script>
tag will not be processed but rendered as a string:
<p [innerHTML]="<script>console.log('fire')</script>"></p>
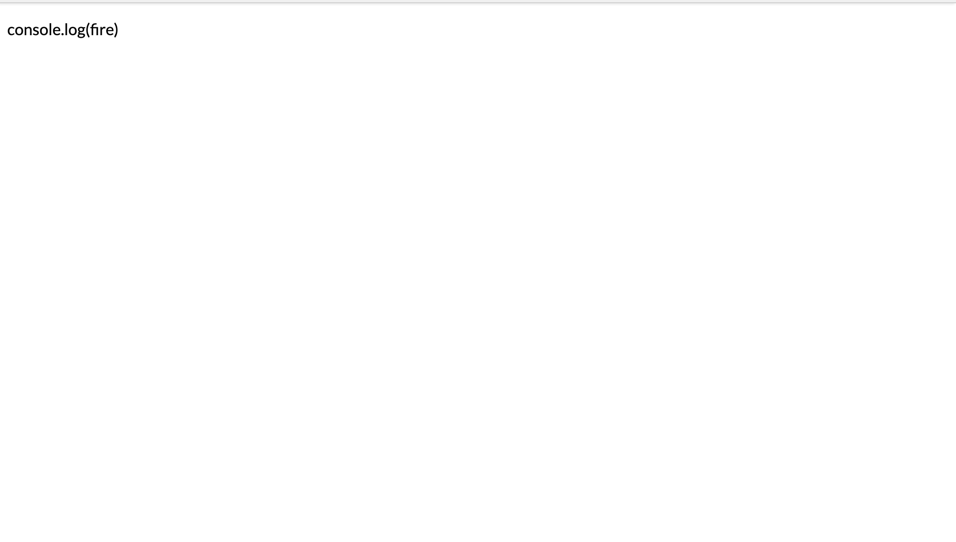
When working with the style attribute, the binding method switches up a bit, you bind directly to the style property involved. If you wanted to update the color
of an element, the following syntax would be used:
//component.html
<h1 [style.color]="color">It's valentines </h1>
In the component end, we’ll define a variable with the color we wish to use:
@Component({
selector: 'app-component',
templateUrl: 'component.html`
})
export class Component{
color = 'indianred';
}
And the element is rendered on the page like the screenshot below:
When the need arises to bind data to multiple style properties, we have to bind them multiple times to each targeted property. The above element’s font size and opacity can be updated using the method shown in the example below:
<h1 [style.color]="color" [style.font-size]="fontSize" [style.opacity]="opacity">It's valentines </h1>
… and then declare the variables in the component:
// component.ts
@Component({
selector: 'app-component',
templateUrl: 'component.html`
})
export class Component{
color = 'indianred';
fontSize = '15px';
opacity = 0.7;
}
When setting the style properties, we used a syntax similar to that of CSS, where properties are using the dash notation. The camel case notation can be used in the same situation in a similar way. Values like font-size
will presented
as fontSize
:
<h1 [style.font-size]="fontSize">It's valentines </h1>
Another method of dealing with the style attribute in Angular is Angular’s own directive ngStyle. This can be used to set multiple style properties using
an object containing key value pairs, with the keys representing the style property and value representing the value to be set. We can rewrite the example above using the ngStyle
directive:
<h1 [ngStyle]="styles">It's valentines </h1>
And then we’ll create an object containing key value pairs.
@Component({
selector: 'app-component',
templateUrl: 'component.html`
})
export class Component{
styles = {
color: 'indianred',
fontSize: '15px',
opacity: 0.7,
}
}
To bind the CSS classes on an element, we can define a variable with the the list of classes within a string field in the component, which is then assigned to the class property. The following snippet sets a class to the heading element:
<h1 [class]="valentines">It's valentines </h1>
We can create this field property in the component :
@Component({
selector: 'app-component',
templateUrl: 'component.html`
})
export class Component{
valentines = 'valentine-class text-bold';
styles = {
color: 'indianred',
fontSize: '15px',
opacity: 0.7,
}
}
The ngClass
Angular directive also exists and can be used interchangeably with the class
attribute binding. In the example above, if we replace [class]
with [ngClass]
, it’ll bear the same results.
When using property binding, it is important to remember the following guidelines:
Understanding Angular’s data binding types is important when building Angular applications. With this knowledge, you can properly utilize the data binding method most suitable for the task at hand. The two data binding methods looked at in this article are both one-way data binding methods and are used to send data from the component layer to the view template. It doesn’t work the other way around. I hope this article broadens your knowledge of data binding in Angular, as data binding is very important and can’t be avoided when working with frontend frameworks.
Update: Since writing this post, we have published a comprehensive 8-part series on Angular Data Binding. Find them here:
For More Info on Building Great Web Apps
Want to learn more about creating great web apps? It all starts out with Kendo UI—the complete UI component library that allows you to quickly build high-quality, responsive apps. It includes everything you need, from grids and charts to dropdowns and gauges.
Learn More about Kendo UI Get a Free Trial of Kendo UIChris Nwamba is a Senior Developer Advocate at AWS focusing on AWS Amplify. He is also a teacher with years of experience building products and communities.