I need to save images using FileExplorer into SQL database, I created a classDataServer.cs and DBContentProvider.cs, I placed them in the App_Code folder. The class DataServer.cs I changed the name of the table Items in Pictures and name of the column ItemID PictureID. Everything else remained unchanged.
The Handler created I changed only the ConnectionString.
After running everything works, but the image is not stored in the database.
Where we make mistaken?
The Handler created I changed only the ConnectionString.
After running everything works, but the image is not stored in the database.
Where we make mistaken?
18 Answers, 1 is accepted
0
Hi Patrik,
I am not quite sure what may be causing the problem. A possible reason for such problem is the table and field were not changed in all SQL commands. Could you please verify if this is not the case? The DataServer methods responsible for storing an item in the database are : ReplaceItemContent(), CreateItem().
To be able to provide more to the point answer we will need to examine and debug the content provider locally. Could you please open a formal support ticket and provide a sample project with the custom content provider implemented?
All the best,
Dobromir
the Telerik team
I am not quite sure what may be causing the problem. A possible reason for such problem is the table and field were not changed in all SQL commands. Could you please verify if this is not the case? The DataServer methods responsible for storing an item in the database are : ReplaceItemContent(), CreateItem().
To be able to provide more to the point answer we will need to examine and debug the content provider locally. Could you please open a formal support ticket and provide a sample project with the custom content provider implemented?
All the best,
Dobromir
the Telerik team
Register for the Q2 2011 What's New Webinar Week. Mark your calendar for the week starting July 18th and book your seat for a walk through of all the exciting stuff we will ship with the new release!
0

Patrik
Top achievements
Rank 2
answered on 08 Jul 2011, 03:36 AM
Columns in the SQL tables have the same names as column names in the linkDataServer.cs class.
Example of a set of project
DataServer.cs
DBContentProvider.cs
Example of a set of project
DataServer.cs
using
System;
using
System.Collections;
using
System.Data;
using
System.Data.SqlClient;
namespace
TrazzyProject
{
/// <summary>
/// Retrieves data from the database and serves content to the DBContentProvider
/// </summary>
public
class
DataServer
{
private
string
_connectionString =
null
;
private
string
ConnectionString
{
get
{
return
_connectionString;
}
}
private
SqlConnection _connection;
private
SqlConnection Connection
{
get
{
if
(_connection ==
null
)
{
_connection =
new
SqlConnection(ConnectionString);
}
return
_connection;
}
}
private
DataTable _data;
private
DataTable Data
{
get
{
if
(_data ==
null
)
{
SqlDataAdapter adapter =
new
SqlDataAdapter(
"SELECT PictureID, Name, ParentID, MimeType, IsDirectory, Size FROM Pictures ORDER BY PictureID"
, Connection);
Connection.Open();
_data =
new
DataTable();
adapter.Fill(_data);
CloseConnection();
}
return
_data;
}
}
/// <summary>
/// Creates an instance of the DataServer class
/// </summary>
/// <param name="connectionString"></param>
public
DataServer(
string
connectionString)
{
_connectionString = connectionString;
}
private
void
CloseConnection()
{
if
(Connection.State != ConnectionState.Closed)
{
Connection.Close();
}
}
private
int
GetItemId(
string
path)
{
DataRow itemRow = GetItemRow(path);
if
(itemRow ==
null
)
{
return
-1;
}
return
(
int
)itemRow[
"PictureID"
];
}
public
DataRow[] GetChildDirectoryRows(
string
path)
{
return
Data.Select(
string
.Format(
"ParentID={0} AND IsDirectory"
, GetItemId(path)));
}
public
DataRow[] GetChildFileRows(
string
path)
{
return
Data.Select(
string
.Format(
"ParentID={0} AND NOT IsDirectory"
, GetItemId(path)));
}
public
DataRow GetItemRow(
string
path)
{
if
(path.EndsWith(
"/"
))
{
path = path.Substring(0, path.Length - 1);
}
string
[] names = path.Split(
'/'
);
//Start search in root;
DataRow searchedRow =
null
;
int
pictureId = 0;
for
(
int
i = 0; i < names.Length; i++)
{
string
name = names[i];
DataRow[] rows = Data.Select(
string
.Format(
"Name='{0}' AND (ParentID={1} OR {1}=0)"
, name.Replace(
"'"
,
"''"
), pictureId));
if
(rows.Length == 0)
{
return
null
;
}
searchedRow = rows[0];
pictureId = (
int
)searchedRow[
"PictureID"
];
}
return
searchedRow;
}
public
byte
[] GetContent(
string
path)
{
int
pictureId = GetItemId(path);
if
(pictureId <= 0)
{
return
null
;
}
SqlCommand command =
new
SqlCommand(
"SELECT Content FROM Pictures WHERE PictureID = @PictureID"
, Connection);
command.Parameters.Add(
new
SqlParameter(
"@PictureID"
, pictureId));
Connection.Open();
byte
[] returnValue = (
byte
[])command.ExecuteScalar();
CloseConnection();
return
returnValue;
}
public
void
CreateItem(
string
name,
string
path,
string
mimeType,
bool
isDirectory,
long
size,
byte
[] content)
{
int
parentId = GetItemId(path);
if
(parentId < 0)
{
return
;
}
SqlCommand command =
new
SqlCommand(
"INSERT INTO Pictures ([Name], ParentId, MimeType, IsDirectory, [Size], Content) VALUES (@Name, @ParentId, @MimeType, @IsDirectory, @Size, @Content)"
, Connection);
command.Parameters.Add(
new
SqlParameter(
"@Name"
, name));
command.Parameters.Add(
new
SqlParameter(
"@ParentId"
, parentId));
command.Parameters.Add(
new
SqlParameter(
"@MimeType"
, mimeType));
command.Parameters.Add(
new
SqlParameter(
"@IsDirectory"
, isDirectory));
command.Parameters.Add(
new
SqlParameter(
"@Size"
, size));
command.Parameters.Add(
new
SqlParameter(
"@Content"
, content));
Connection.Open();
command.ExecuteNonQuery();
CloseConnection();
_data =
null
;
}
public
void
DeleteItem(
string
path)
{
//TODO: cascade delete when removing a non-empty folder
int
pictureId = GetItemId(path);
if
(pictureId <= 0)
{
return
;
}
SqlCommand command =
new
SqlCommand(
"DELETE FROM Pictures WHERE PictureID=@PictureID"
, Connection);
command.Parameters.Add(
new
SqlParameter(
"@PictureID"
, pictureId));
Connection.Open();
command.ExecuteNonQuery();
CloseConnection();
_data =
null
;
}
public
DataRow[] GetAllDirectoryRows(
string
path)
{
DataRow rootRow = GetItemRow(path);
if
(rootRow ==
null
)
{
return
new
DataRow[] { };
}
ArrayList allDirectoryRows =
new
ArrayList();
allDirectoryRows.Add(rootRow);
FillChildDirectoryRows((
int
)rootRow[
"PictureID"
], allDirectoryRows);
return
(DataRow[])allDirectoryRows.ToArray(
typeof
(DataRow));
}
private
void
FillChildDirectoryRows(
int
parentId, ArrayList toFill)
{
DataRow[] childRows = Data.Select(
string
.Format(
"ParentID={0} AND IsDirectory"
, parentId));
foreach
(DataRow childRow
in
childRows)
{
toFill.Add(childRow);
FillChildDirectoryRows((
int
)childRow[
"PictureID"
], toFill);
}
}
public
string
GetItemPath(DataRow row)
{
if
(row[
"ParentID"
]
is
DBNull)
{
return
string
.Format(
"{0}/"
, row[
"Name"
]);
}
int
parentId = (
int
)row[
"ParentID"
];
DataRow[] parents = Data.Select(
string
.Format(
"PictureID={0}"
, parentId));
if
(parents.Length == 0)
{
return
string
.Format(
"/{0}"
, row[
"Name"
]);
}
return
GetItemPath(parents[0]) +
string
.Format(
"{0}/"
, row[
"Name"
]);
}
public
void
ReplaceItemContent(
string
path,
byte
[] content)
{
int
pictureId = GetItemId(path);
if
(pictureId < 0)
{
return
;
}
SqlCommand command =
new
SqlCommand(
"UPDATE Pictures SET Content=@Content WHERE PictureID=@PictureID"
, Connection);
command.Parameters.Add(
new
SqlParameter(
"@Content"
, content));
command.Parameters.Add(
new
SqlParameter(
"@PictureID"
, pictureId));
Connection.Open();
command.ExecuteNonQuery();
CloseConnection();
}
public
void
UpdateItemPath(
string
path,
string
newName,
int
newParentId)
{
int
pictureId = GetItemId(path);
if
(pictureId < 0)
{
return
;
}
SqlCommand command =
new
SqlCommand(
"UPDATE Pictures SET [Name]=@Name, ParentId=@ParentId WHERE PictureID=@PictureID"
, Connection);
command.Parameters.Add(
new
SqlParameter(
"@Name"
, newName));
command.Parameters.Add(
new
SqlParameter(
"@ParentId"
, newParentId));
command.Parameters.Add(
new
SqlParameter(
"@PictureID"
, pictureId));
Connection.Open();
command.ExecuteNonQuery();
CloseConnection();
}
/// <summary>
/// Checks whether the item(file or folder) exists on the server
/// </summary>
/// <param name="pathToItem">Path to file or folder</param>
/// <returns></returns>
public
bool
IsItemExists(
string
pathToItem)
{
DataRow row = GetItemRow(pathToItem);
return
row !=
null
;
}
}
}
DBContentProvider.cs
using
System;
using
System.Data;
using
System.Drawing;
using
System.Drawing.Imaging;
using
System.IO;
using
System.Configuration;
using
System.Web;
using
System.Web.Security;
using
System.Web.UI;
using
System.Web.UI.WebControls;
using
System.Web.UI.WebControls.WebParts;
using
System.Web.UI.HtmlControls;
using
Telerik.Web.UI.Widgets;
using
System.Collections.Generic;
using
System.Collections;
namespace
TrazzyProject
{
//FileBrowserContentProvider
public
class
DBContentProvider : FileBrowserContentProvider
{
private
DataServer _dataServer;
private
DataServer DataServer
{
get
{
if
(Object.Equals(_dataServer,
null
))
{
_dataServer =
new
DataServer(ConfigurationManager.ConnectionStrings[
"SampleConnection"
].ConnectionString);
}
return
_dataServer;
}
}
private
string
_itemHandlerPath;
private
string
ItemHandlerPath
{
get
{
return
_itemHandlerPath;
}
}
public
DBContentProvider(HttpContext context,
string
[] searchPatterns,
string
[] viewPaths,
string
[] uploadPaths,
string
[] deletePaths,
string
selectedUrl,
string
selectedItemTag)
:
base
(context, searchPatterns, viewPaths, uploadPaths, deletePaths, selectedUrl, selectedItemTag)
{
_itemHandlerPath = ConfigurationManager.AppSettings[
"TrazzyProject.ItemHandler"
];
if
(_itemHandlerPath.StartsWith(
"~/"
))
{
_itemHandlerPath = HttpContext.Current.Request.ApplicationPath + _itemHandlerPath.Substring(1);
}
}
private
string
GetItemUrl(
string
virtualItemPath)
{
string
escapedPath = Context.Server.UrlEncode(virtualItemPath);
return
string
.Format(
"{0}?path={1}"
, ItemHandlerPath, escapedPath);
}
private
string
ExtractPath(
string
itemUrl)
{
if
(itemUrl ==
null
)
{
return
string
.Empty;
}
if
(itemUrl.StartsWith(_itemHandlerPath))
{
return
itemUrl.Substring(GetItemUrl(
string
.Empty).Length);
}
return
itemUrl;
}
private
string
GetName(
string
path)
{
if
(String.IsNullOrEmpty(path) || path ==
"/"
)
{
return
string
.Empty;
}
path = VirtualPathUtility.RemoveTrailingSlash(path);
return
path.Substring(path.LastIndexOf(
'/'
) + 1);
}
private
string
GetDirectoryPath(
string
path)
{
return
path.Substring(0, path.LastIndexOf(
'/'
) + 1);
}
private
bool
IsChildOf(
string
parentPath,
string
childPath)
{
return
childPath.StartsWith(parentPath);
}
private
string
CombinePath(
string
path1,
string
path2)
{
if
(path1.EndsWith(
"/"
))
{
return
string
.Format(
"{0}{1}"
, path1, path2);
}
if
(path1.EndsWith(
"\\"
))
{
path1 = path1.Substring(0, path1.Length - 1);
}
return
string
.Format(
"{0}/{1}"
, path1, path2);
}
private
DirectoryItem[] GetChildDirectories(
string
path)
{
List<DirectoryItem> directories =
new
List<DirectoryItem>();
try
{
DataRow[] childRows = DataServer.GetChildDirectoryRows(path);
int
i = 0;
while
(i < childRows.Length)
{
DataRow childRow = childRows[i];
string
name = childRow[
"Name"
].ToString();
string
itemFullPath = VirtualPathUtility.AppendTrailingSlash(CombinePath(path, name));
DirectoryItem newDirItem =
new
DirectoryItem(name,
string
.Empty,
itemFullPath,
string
.Empty,
GetPermissions(itemFullPath),
null
,
// The files are added in ResolveDirectory()
null
// Directories are added in ResolveRootDirectoryAsTree()
);
directories.Add(newDirItem);
i = i + 1;
}
return
directories.ToArray();
}
catch
(Exception)
{
return
new
DirectoryItem[] { };
}
}
private
FileItem[] GetChildFiles(
string
_path)
{
try
{
DataRow[] childRows = DataServer.GetChildFileRows(_path);
List<FileItem> files =
new
List<FileItem>();
for
(
int
i = 0; i < childRows.Length; i++)
{
DataRow childRow = childRows[i];
string
name = childRow[
"Name"
].ToString();
if
(IsExtensionAllowed(System.IO.Path.GetExtension(name)))
{
string
itemFullPath = CombinePath(_path, name);
FileItem newFileItem =
new
FileItem(name,
Path.GetExtension(name),
(
int
)childRow[
"Size"
],
itemFullPath,
GetItemUrl(itemFullPath),
string
.Empty,
GetPermissions(itemFullPath)
);
files.Add(newFileItem);
}
}
return
files.ToArray();
}
catch
(Exception)
{
return
new
FileItem[] { };
}
}
private
bool
IsExtensionAllowed(
string
extension)
{
return
Array.IndexOf(SearchPatterns,
"*.*"
) >= 0 || Array.IndexOf(SearchPatterns,
"*"
+ extension.ToLower()) >= 0;
}
/// <summary>
/// Checks Upload permissions
/// </summary>
/// <param name="path">Path to an item</param>
/// <returns></returns>
private
bool
HasUploadPermission(
string
path)
{
foreach
(
string
uploadPath
in
this
.UploadPaths)
{
if
(path.StartsWith(uploadPath, StringComparison.CurrentCultureIgnoreCase))
{
return
true
;
}
}
return
false
;
}
/// <summary>
/// Checks Delete permissions
/// </summary>
/// <param name="path">Path to an item</param>
/// <returns></returns>
private
bool
HasDeletePermission(
string
path)
{
foreach
(
string
deletePath
in
this
.DeletePaths)
{
if
(path.StartsWith(deletePath, StringComparison.CurrentCultureIgnoreCase))
{
return
true
;
}
}
return
false
;
}
/// <summary>
/// Returns the permissions for the provided path
/// </summary>
/// <param name="pathToItem">Path to an item</param>
/// <returns></returns>
private
PathPermissions GetPermissions(
string
pathToItem)
{
PathPermissions permission = PathPermissions.Read;
permission = HasUploadPermission(pathToItem) ? permission | PathPermissions.Upload : permission;
permission = HasDeletePermission(pathToItem) ? permission | PathPermissions.Delete : permission;
return
permission;
}
/// <summary>
/// Loads a root directory with given path, where all subdirectories
/// contained in the SelectedUrl property are loaded
/// </summary>
/// <remarks>
/// The ImagesPaths, DocumentsPaths, etc properties of RadEditor
/// allow multiple root items to be specified, separated by comma, e.g.
/// Photos,Paintings,Diagrams. The FileBrowser class calls the
/// ResolveRootDirectoryAsTree method for each of them.
/// </remarks>
/// <param name="path">the root directory path, passed by the FileBrowser</param>
/// <returns>The root DirectoryItem or null if such does not exist</returns>
public
override
DirectoryItem ResolveRootDirectoryAsTree(
string
path)
{
DirectoryItem returnValue =
new
DirectoryItem(GetName(path),
GetDirectoryPath(path),
path,
string
.Empty,
GetPermissions(path),
null
,
// The files are added in ResolveDirectory()
GetChildDirectories(path));
return
returnValue;
}
public
override
DirectoryItem ResolveDirectory(
string
path)
{
DirectoryItem[] directories = GetChildDirectories(path);
DirectoryItem returnValue =
new
DirectoryItem(GetName(path),
VirtualPathUtility.AppendTrailingSlash(GetDirectoryPath(path)),
path,
string
.Empty,
GetPermissions(path),
GetChildFiles(path),
null
// Directories are added in ResolveRootDirectoryAsTree()
);
return
returnValue;
}
public
override
string
GetFileName(
string
url)
{
return
GetName(url);
}
public
override
string
GetPath(
string
url)
{
return
GetDirectoryPath(ExtractPath(RemoveProtocolNameAndServerName(url)));
}
public
override
Stream GetFile(
string
url)
{
byte
[] content = DataServer.GetContent(ExtractPath(RemoveProtocolNameAndServerName(url)));
if
(!Object.Equals(content,
null
))
{
return
new
MemoryStream(content);
}
return
null
;
}
public
override
string
StoreBitmap(Bitmap bitmap,
string
url, ImageFormat format)
{
string
newItemPath = ExtractPath(RemoveProtocolNameAndServerName(url));
string
name = GetName(newItemPath);
string
_path = GetPath(newItemPath);
string
tempFilePath = System.IO.Path.GetTempFileName();
bitmap.Save(tempFilePath);
byte
[] content;
using
(FileStream inputStream = File.OpenRead(tempFilePath))
{
long
size = inputStream.Length;
content =
new
byte
[size];
inputStream.Read(content, 0, Convert.ToInt32(size));
}
if
(File.Exists(tempFilePath))
{
File.Delete(tempFilePath);
}
DataServer.CreateItem(name, _path,
"image/gif"
,
false
, content.Length, content);
return
string
.Empty;
}
public
override
string
MoveFile(
string
path,
string
newPath)
{
try
{
bool
destFileExists =
this
.DataServer.IsItemExists(newPath);
if
(destFileExists)
return
"A file with the same name exists in the destination folder"
;
string
newFileName = GetName(newPath);
string
destinationDirPath = newPath.Substring(0, newPath.Length - newFileName.Length);
if
(destinationDirPath.Length == 0)
{
destinationDirPath = path.Substring(0, path.LastIndexOf(
"/"
));
}
// destination directory row
DataRow newPathRow = DataServer.GetItemRow(destinationDirPath);
DataServer.UpdateItemPath(path, newFileName, (
int
)newPathRow[
"PictureID"
]);
}
catch
(Exception e)
{
return
e.Message;
}
return
string
.Empty;
}
public
override
string
MoveDirectory(
string
path,
string
newPath)
{
if
(newPath.EndsWith(
"/"
)) newPath = newPath.Remove(newPath.Length - 1, 1);
bool
destFileExists =
this
.DataServer.IsItemExists(newPath);
if
(destFileExists)
return
"A directory with the same name exists in the destination folder"
;
return
MoveFile(path, newPath);
}
public
override
string
CopyFile(
string
path,
string
newPath)
{
try
{
bool
destFileExists =
this
.DataServer.IsItemExists(newPath);
if
(destFileExists)
return
"A file with the same name exists in the destination folder"
;
string
newFileName = GetName(newPath);
string
newFilePath = newPath.Substring(0, newPath.Length - newFileName.Length);
if
(newFilePath.Length == 0)
{
newFilePath = path.Substring(0, path.LastIndexOf(
"/"
));
}
DataRow oldPathRow = DataServer.GetItemRow(path);
DataServer.CreateItem(newFileName, newFilePath, (
string
)oldPathRow[
"MimeType"
], (
bool
)oldPathRow[
"IsDirectory"
], (
int
)oldPathRow[
"Size"
], DataServer.GetContent(path));
if
((
bool
)oldPathRow[
"IsDirectory"
])
{
//copy all child items of the folder as well
FileItem[] files = GetChildFiles(path);
foreach
(FileItem childFile
in
files)
{
CopyFile(childFile.Tag, CombinePath(newPath, childFile.Name));
}
//copy all child folders as well
DirectoryItem[] subFolders = GetChildDirectories(path);
foreach
(DirectoryItem subFolder
in
subFolders)
{
CopyFile(subFolder.Tag, CombinePath(newPath, subFolder.Name));
}
}
}
catch
(Exception e)
{
return
e.Message;
}
return
string
.Empty;
}
public
override
string
CopyDirectory(
string
path,
string
newPath)
{
string
[] pathParts = path.Split(
new
char
[] {
'/'
}, StringSplitOptions.RemoveEmptyEntries);
if
(pathParts.Length > 0)
{
string
fullNewPath = CombinePath(newPath, pathParts[pathParts.Length - 1]);
bool
destFileExists =
this
.DataServer.IsItemExists(fullNewPath);
if
(destFileExists)
return
"A file with the same name exists in the destination folder"
;
return
CopyFile(path, fullNewPath);
}
else
return
"Old path is invalid"
;
}
public
override
string
StoreFile(Telerik.Web.UI.UploadedFile file,
string
path,
string
name,
params
string
[] arguments)
{
int
fileLength = Convert.ToInt32(file.InputStream.Length);
byte
[] content =
new
byte
[fileLength];
file.InputStream.Read(content, 0, fileLength);
string
fullPath = CombinePath(path, name);
if
(!Object.Equals(DataServer.GetItemRow(fullPath),
null
))
{
DataServer.ReplaceItemContent(fullPath, content);
}
else
{
DataServer.CreateItem(name, path, file.ContentType,
false
, fileLength, content);
}
return
string
.Empty;
}
public
override
string
DeleteFile(
string
path)
{
DataServer.DeleteItem(path);
return
string
.Empty;
}
public
override
string
DeleteDirectory(
string
path)
{
DataServer.DeleteItem(path);
return
string
.Empty;
}
public
override
string
CreateDirectory(
string
path,
string
name)
{
DataServer.CreateItem(name, path,
string
.Empty,
true
, 0,
new
byte
[0]);
return
string
.Empty;
}
public
override
bool
CanCreateDirectory
{
get
{
return
true
;
}
}
}
}
0
Hi Patrik,
I tested the provided ContentProvider and DataServer classes and everything is working as expected on my side. In my previous answer I asked for fully runnable project so we can examine the database as well. Could you please verify that the Content field is the correct type - one able to store files (Binary, Varbinary, Image)?
In addition, you can debug the ReplaceItemContent() / CreateItem() methods to see if the SQL Query is executed correctly - ExecuteNonQuery() should return the number of affected rows, thus when executed the returned value should be 1.
Regards,
Dobromir
the Telerik team
I tested the provided ContentProvider and DataServer classes and everything is working as expected on my side. In my previous answer I asked for fully runnable project so we can examine the database as well. Could you please verify that the Content field is the correct type - one able to store files (Binary, Varbinary, Image)?
In addition, you can debug the ReplaceItemContent() / CreateItem() methods to see if the SQL Query is executed correctly - ExecuteNonQuery() should return the number of affected rows, thus when executed the returned value should be 1.
Regards,
Dobromir
the Telerik team
Register for the Q2 2011 What's New Webinar Week. Mark your calendar for the week starting July 18th and book your seat for a walk through of all the exciting stuff we will ship with the new release!
0

Rohan
Top achievements
Rank 1
answered on 11 May 2012, 01:01 PM
Hi Dobromir,
I am implement all the class and the same table structure as provided ,but i am not able to get tree structure as per table's record .
I have Multiple level of folder and file structure in database table . so how can i do this ,please help us .
I am implement all the class and the same table structure as provided ,but i am not able to get tree structure as per table's record .
I have Multiple level of folder and file structure in database table . so how can i do this ,please help us .
0
Hi Rohan,
The provided information is not enough to determine the exact cause of the issue, could you please describe in more details what do you mean by "I have Multiple level of folder and file structure in database table" ? Could you please open formal support ticket and provide a sample fully runnable project along with sample database reproducing the issue so we can examine and debug it locally?
Regards,
Dobromir
the Telerik team
The provided information is not enough to determine the exact cause of the issue, could you please describe in more details what do you mean by "I have Multiple level of folder and file structure in database table" ? Could you please open formal support ticket and provide a sample fully runnable project along with sample database reproducing the issue so we can examine and debug it locally?
Regards,
Dobromir
the Telerik team
If you want to get updates on new releases, tips and tricks and sneak peeks at our product labs directly from the developers working on the RadControls for ASP.NET AJAX, subscribe to their blog feed now.
0

Rohan
Top achievements
Rank 1
answered on 21 May 2012, 10:27 AM
HI Dobromir
Thanks for replay,
I want to show the various levels of folder and files , please refer screen shot.
0

Rohan
Top achievements
Rank 1
answered on 21 May 2012, 03:31 PM
Hi Dobromir,
I solve my previous post problem. now second problem is - I want to insert two button on file explore which renames the nodes of rad file explore tree . Nodes names are logically different than names contains on database table , how can i do this.
I solve my previous post problem. now second problem is - I want to insert two button on file explore which renames the nodes of rad file explore tree . Nodes names are logically different than names contains on database table , how can i do this.
0
Hi Rohan,
You can modify the names of the folders by modifying the Name parameter of the DirectoryItems returned by the ResolveRootDirectoryAsTree() method of the content provider, e.g.:
More detailed information on how RadFileExplorer content provider's working is available in the following help article:
Using custom FileBrowserContentProvider
Kind regards,
Dobromir
the Telerik team
You can modify the names of the folders by modifying the Name parameter of the DirectoryItems returned by the ResolveRootDirectoryAsTree() method of the content provider, e.g.:
public
override
DirectoryItem ResolveRootDirectoryAsTree(
string
path)
{
var orgDir =
base
.ResolveRootDirectoryAsTree(path);
orgDir.Name = orgDir.Name +
new
Random().Next().ToString();
//modify the name of the current folder
//modify the name of the subfolders
foreach
(var dir
in
orgDir.Directories)
{
dir.Name = dir.Name +
new
Random().Next().ToString();
}
return
orgDir;
}
More detailed information on how RadFileExplorer content provider's working is available in the following help article:
Using custom FileBrowserContentProvider
Kind regards,
Dobromir
the Telerik team
If you want to get updates on new releases, tips and tricks and sneak peeks at our product labs directly from the developers working on the RadControls for ASP.NET AJAX, subscribe to their blog feed now.
0

Rohan
Top achievements
Rank 1
answered on 30 May 2012, 10:57 AM
Hi Dobromir
Thanks for Replay ......
Thanks for Replay ......
0

Rohan
Top achievements
Rank 1
answered on 31 May 2012, 10:20 AM
Hi Dobromir,
Thanks for replay ..
I am able to rename the directory on File Explore . But have one different scenario as - i have two button on File Explorer tool bar one for User Name and other for First name and last name. When user click on UserName Button Treeview show the directory name as UserName and when user click on First name and Last name Treeview show the directory name as First Name and Last name.
Problem is - When User Click in First Name and Last Name Button it effects only to Rad Grid (Show the first name and Last Name) but it does not effect to tree view tree view not showing as refreshed data .(may be tree view is not getting refreshing) how can i do this .......
Thanks for replay ..
I am able to rename the directory on File Explore . But have one different scenario as - i have two button on File Explorer tool bar one for User Name and other for First name and last name. When user click on UserName Button Treeview show the directory name as UserName and when user click on First name and Last name Treeview show the directory name as First Name and Last name.
Problem is - When User Click in First Name and Last Name Button it effects only to Rad Grid (Show the first name and Last Name) but it does not effect to tree view tree view not showing as refreshed data .(may be tree view is not getting refreshing) how can i do this .......
0

Rohan
Top achievements
Rank 1
answered on 31 May 2012, 10:29 AM
Hi Dobromir,
Problem is solved ...
Problem is solved ...
0

Rohan
Top achievements
Rank 1
answered on 31 May 2012, 02:17 PM
0
Hi Rohan,
To avoid full postback for uploading file, you need to enable the AsyncUpload for RadFileExplorer. Please take a look at the following live demo for more detailed information:
FileExplorer / AsyncUpload
All the best,
Dobromir
the Telerik team
To avoid full postback for uploading file, you need to enable the AsyncUpload for RadFileExplorer. Please take a look at the following live demo for more detailed information:
FileExplorer / AsyncUpload
All the best,
Dobromir
the Telerik team
If you want to get updates on new releases, tips and tricks and sneak peeks at our product labs directly from the developers working on the RadControls for ASP.NET AJAX, subscribe to their blog feed now.
0

Rohan
Top achievements
Rank 1
answered on 02 Jun 2012, 10:14 AM
Hi Dobromir
Thanks for replay ,
this is syntax i am using , still it cause postback to upload functionality of file exchange .
<telerik:RadFileExplorer runat="server" ID="FileExplorer1" Width="100%" Height="400" CssClass="rfeFocus"
AllowPaging="true" PageSize="0" OnClientItemSelected="OnClientItemSelected" EnableAsyncUpload="true">
<Configuration
DeletePaths="~/FileExplorer/Examples/Default/Images" />
<KeyboardShortcuts
FocusFileExplorer="Ctrl+f2"
FocusToolBar="Shift+1"
FocusAddressBar="Shift+2"
FocusTreeView="Shift+3"
FocusGrid="Shift+4"
FocusGridPagingSlider="Shift+5"
UploadFile="Ctrl+u"
Back="Ctrl+k"
Forward="Ctrl+l"
NewFolder="Ctrl+n"
Refresh="Ctrl+f3"
ContextMenu="Shift+m" />
</telerik:RadFileExplorer>
i am also try with Update panel of ajax and RadAjaxPanel of telelrik ..
Thanks for replay ,
this is syntax i am using , still it cause postback to upload functionality of file exchange .
<telerik:RadFileExplorer runat="server" ID="FileExplorer1" Width="100%" Height="400" CssClass="rfeFocus"
AllowPaging="true" PageSize="0" OnClientItemSelected="OnClientItemSelected" EnableAsyncUpload="true">
<Configuration
DeletePaths="~/FileExplorer/Examples/Default/Images" />
<KeyboardShortcuts
FocusFileExplorer="Ctrl+f2"
FocusToolBar="Shift+1"
FocusAddressBar="Shift+2"
FocusTreeView="Shift+3"
FocusGrid="Shift+4"
FocusGridPagingSlider="Shift+5"
UploadFile="Ctrl+u"
Back="Ctrl+k"
Forward="Ctrl+l"
NewFolder="Ctrl+n"
Refresh="Ctrl+f3"
ContextMenu="Shift+m" />
</telerik:RadFileExplorer>
i am also try with Update panel of ajax and RadAjaxPanel of telelrik ..
0
Hi Rohan,
Which version of RadControls for ASP.NET AJAX you are using in your application? If you are using version prior 2011.2.915 there was a bug in RadFileExplorer causing the control to postback after upload. If this is the case, I would recommend you to upgrade your version of RadControls to the latest available (currently 2012.1.411).
Kind regards,
Dobromir
the Telerik team
Which version of RadControls for ASP.NET AJAX you are using in your application? If you are using version prior 2011.2.915 there was a bug in RadFileExplorer causing the control to postback after upload. If this is the case, I would recommend you to upgrade your version of RadControls to the latest available (currently 2012.1.411).
Kind regards,
Dobromir
the Telerik team
If you want to get updates on new releases, tips and tricks and sneak peeks at our product labs directly from the developers working on the RadControls for ASP.NET AJAX, subscribe to their blog feed now.
0

Rohan
Top achievements
Rank 1
answered on 05 Jun 2012, 03:11 PM
Hi Dobromir,
Thanks for replay ,
once again i come with problem - when ever i click to any command of file explore rad grid of file explorer becomes size as shown in screen shot. my second problem is i want allow user to upload any type of file on file explorer how can i do this?
Once again thanks for you replay and your valuable guidance......
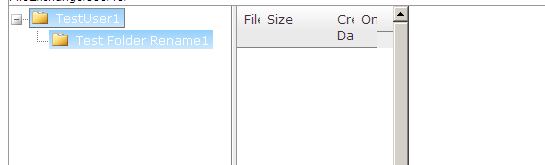
Thanks for replay ,
once again i come with problem - when ever i click to any command of file explore rad grid of file explorer becomes size as shown in screen shot. my second problem is i want allow user to upload any type of file on file explorer how can i do this?
Once again thanks for you replay and your valuable guidance......
0

Rohan
Top achievements
Rank 1
answered on 07 Jun 2012, 11:23 AM
Hi Dobromir,
Now i am using Version 2012.1.215.40 , When ever i click to any command button i can only see the wait symbol ,
as shown in screen shot . how to resolve this problem .
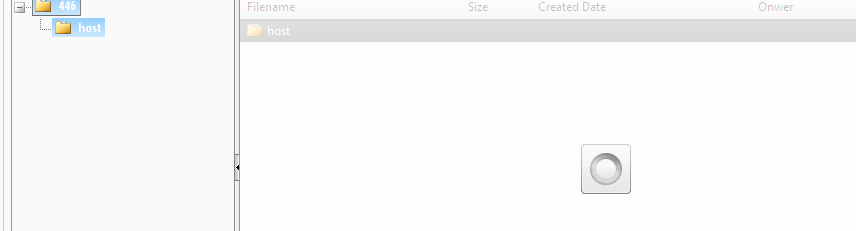
Now i am using Version 2012.1.215.40 , When ever i click to any command button i can only see the wait symbol ,
as shown in screen shot . how to resolve this problem .
0
Hi Rohan,
The provided screenshot is not enough to determine the exact cause of the issue. There are two reasons, that I am aware of, that may be caused the described behavior:
If non of the above is the case, could you please provide sample runnable page reproducing the problem so we can examine it further?
Greetings,
Dobromir
the Telerik team
The provided screenshot is not enough to determine the exact cause of the issue. There are two reasons, that I am aware of, that may be caused the described behavior:
- A server-side error thrown during the callback triggered to populate the grid component of the explorer. If you have assigned custom content provider, I would suggest you to run the page in debug more and set a breakpoints in the ResolveRootDirectoryAsTree() and ResolveDirectory() methods because they are responsible for populating the explorer.
- If an additional AJAX request is triggered and interrupt the callback request by the control. This can happen if RadFileExplorer is ajaxified externally. In general this is not recommended practice, because the explorer's functionality is based on client-side callbacks and they do not work well with additional ajaxification. However, if you need to update the control using AJAX you should note that RadFileExplorer should not be trigger for this request.
If non of the above is the case, could you please provide sample runnable page reproducing the problem so we can examine it further?
Greetings,
Dobromir
the Telerik team
If you want to get updates on new releases, tips and tricks and sneak peeks at our product labs directly from the developers working on the RadControls for ASP.NET AJAX, subscribe to their blog feed now.