Is there away to call the BeginInsert() method on a child grid in a heirarchical grid view? It works fine for me on the master, but I cannot find a way to make it apply to the child grid.
Any input would be appreciated, I couldn't find any forum posts and the documentation isn't really clear on this.
Thanks,
Marty
11 Answers, 1 is accepted
Nothing built-in at the moment. The easiest way to do this is to get all visible GridViewDataControl instances and call BeginInsert(). Here's a quick sample:
private void insertChild_Click(object sender, RoutedEventArgs e) |
{ |
var childControls = this.RadGridView1.ChildrenOfType<GridViewDataControl>().Where(c => !(c is RadGridView)); |
foreach (var control in childControls) |
{ |
control.BeginInsert(); |
} |
} |
I am attaching a sample project for your reference.
Sincerely yours,
Hristo Deshev
the Telerik team
Instantly find answers to your questions on the new Telerik Support Portal.
Check out the tips for optimizing your support resource searches.

Hi Hristo,
Thanks for the reply and example, and sorry for my delayed response...
I downloaded your example, and it works great... however, the same code does not work with my grid. The loop successfully finds the expanded child grids, however, when the beginInsert() method is called, nothing happens. My grid is declared a bit differently than yours, but is still bound to a data source with a property relationship defined... the only difference is that I statically declare my columns so I can have custom headers... take a look and let me know what you think:
XAML:
<telerik:RadGridView Grid.ColumnSpan="2" Name="grdListMgmt" IsReadOnly="False" AutoGenerateColumns="False" ColumnsWidthMode="Auto"> |
<telerik:RadGridView.Columns> |
<telerik:GridViewDataColumn UniqueName="ListManagementID" HeaderText="List ID" IsVisible="False" /> |
<telerik:GridViewDataColumn UniqueName="ListName" HeaderText="List Name" /> |
<telerik:GridViewDataColumn UniqueName="ListHeader" HeaderText="List Header" /> |
</telerik:RadGridView.Columns> |
<telerik:RadGridView.HierarchyChildTemplate> |
<DataTemplate> |
<telerik:GridViewDataControl CellValidating="GrdListMgmtValidation_Validating"/> |
</DataTemplate> |
</telerik:RadGridView.HierarchyChildTemplate> |
</telerik:RadGridView> |
Code Behind (CS):
public ListManagementPage() |
{ |
IsValid = true; |
InitializeComponent(); |
GeneralClient gc = new GeneralClient(); |
_lists = gc.GetListsForEdit(false).ToList(); |
GridViewTableDefinition definition = new GridViewTableDefinition(); |
definition.AutoGenerateFieldDescriptors = false; |
definition.Relation = new PropertyRelation("ListItems"); |
GridViewDataColumn column = new GridViewDataColumn(); |
column.DataMemberBinding = new Binding("ListManagementID"); |
column.DataMemberBinding.Mode = BindingMode.TwoWay; |
column.HeaderText = "List Management ID"; |
column.IsVisible = false; |
definition.FieldDescriptors.Add(column); |
column = new GridViewDataColumn(); |
column.DataMemberBinding = new Binding("ListManagementItemID"); |
column.DataMemberBinding.Mode = BindingMode.TwoWay; |
column.HeaderText = "List Mgmt Item ID"; |
column.IsVisible = false; |
column.IsFilterable = false; |
definition.FieldDescriptors.Add(column); |
column = new GridViewDataColumn(); |
column.DataMemberBinding = new Binding("ItemName"); |
column.DataMemberBinding.Mode = BindingMode.TwoWay; |
column.HeaderText = "Item Name"; |
column.IsFilterable = false; |
definition.FieldDescriptors.Add(column); |
column = new GridViewDataColumn(); |
column.DataMemberBinding = new Binding("ItemValue"); |
column.DataMemberBinding.Mode = BindingMode.TwoWay; |
column.HeaderText = "Item Value"; |
column.IsFilterable = false; |
definition.FieldDescriptors.Add(column); |
grdListMgmt.TableDefinition.ChildTableDefinitions.Add(definition); |
grdListMgmt.ItemsSource = _lists; |
this.grdListMgmt.AddHandler(GridViewDataControl.CellValidatingEvent, |
new EventHandler<GridViewCellValidatingEventArgs>( |
this.GrdListMgmtValidation_Validating)); |
} |
Marty
I have modified the original sample that Hristo has sent you to match your project as closely as possible but the BeginInsert() still works. I believe that your problem is most probably related to the data that is used by the child grids.
Sending us a sample project would really help us get to the bottom of this fast. In case that is not possible could you give us more information about the type of data that you are using -
what is the type of the ListItems collection?
Sincerely yours,
Milan
the Telerik team
Instantly find answers to your questions on the new Telerik Support Portal.
Check out the tips for optimizing your support resource searches.

Thank you for your reply.
Before I send an example, I'll tell you that the datasource is defined as follows:
[DataContract] |
public class cListManagement |
{ |
[DataMember] |
public int? ListManagementID { get; set; } |
[DataMember] |
public string ListName { get; set; } |
[DataMember] |
public string ListHeader { get; set; } |
[DataMember] |
public List<cListManagementItem> ListItems { get; set; } |
} |
and the business object, cListManagementItem is defined as follows:
[DataContract] |
public class cListManagementItem |
{ |
[DataMember] |
public int ListManagementItemID { get; set; } |
[DataMember] |
public int ListManagementID { get; set; } |
[DataMember] |
public string ItemName { get; set; } |
[DataMember] |
public string ItemValue { get; set; } |
} |
They are defined and retrieved through WCF calls to the service they're embedded in.
I hope this helps, if it does not, then, I'll try to work up a sample with these data types.
Thanks again,
Marty
I have managed to figure out what is wrong. The problem is indeed caused by the data that is fed into the grid. Although you have defined the ListItems property like this:
[DataMember] |
public List<cListManagementItem> ListItems { get; set; } |
Luckily there is an easy solution - when adding the service reference you can open the advanced options dialog and manually choose collection type.
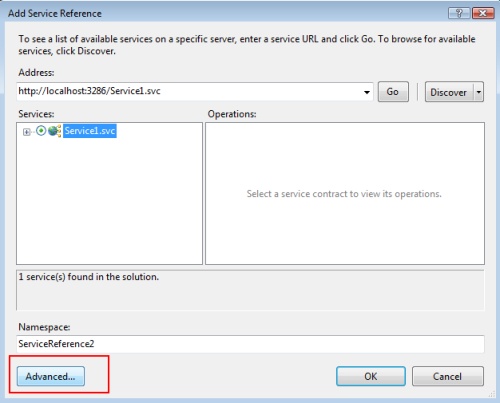
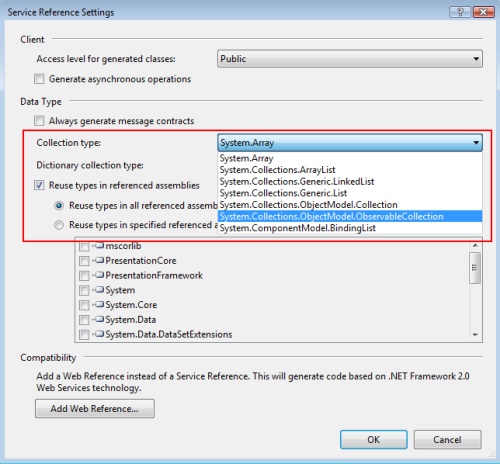
Choosing List, Collection, ObservableCollection or BindingList should solve the problem.
I am also sending you my test project.
Hope this helps
Kind regards,
Milan
the Telerik team
Instantly find answers to your questions on the new Telerik Support Portal.
Check out the tips for optimizing your support resource searches.

Milan,
Thank you for your help. I realized what you were going to say shortly after I posted my last post... I'm glad we came to the same conclusion. Therefore, I was able to work around this by creating a local type with an ObservableCollection<T> object rather than an array and used it to bind to the list instead of the type from the WCF service.
Now, this works... however, I have one more dilemma. The code provided calls BeginInsert() on ALL expanded child grids, however, I only want to call it on the one whose parent row is selected, if that makes sense. Is there an easy way to do this?
Thanks again,
Marty
That is actually quite easy to do. Just modify the insertChild_Click event handler:
private void insertChild_Click(object sender, RoutedEventArgs e) |
{ |
ExpandableDataRecord dataRecord = this.grdListMgmt.SelectedRecord as ExpandableDataRecord; |
var hierarchyRow = (GridViewExpandableRow)this.grdListMgmt.ItemsControl.ItemsGenerator.ContainerFromItem(dataRecord); |
if(hierarchyRow != null) |
hierarchyRow.ChildDataControls[0].BeginInsert(); |
} |
Kind regards,
Milan
the Telerik team
Instantly find answers to your questions on the new Telerik Support Portal.
Check out the tips for optimizing your support resource searches.

Marty
It is great to hear that everything is working now.
Sincerely yours,
Milan
the Telerik team
Instantly find answers to your questions on the new Telerik Support Portal.
Check out the tips for optimizing your support resource searches.

is it possible to define GridViewTableDefinition in XAML, so it is not necessary to define it in code behind?
Thanks for replay,
Tomas
Unfortunately defining GridViewTableDefinition in XAML is currently not supported. We are working on providing such support but it is still unclear when it will be available.
Greetings,
Milan
the Telerik team
Instantly find answers to your questions on the new Telerik Support Portal.
Check out the tips for optimizing your support resource searches.