RadChart allows you to programmatically filter its data using FilterDescriptors as demonstrated in our help topic. However there are scenarios where you would like to have more complex filtering. For a sample scenario where a Chart is drawn according to the filtered RadGridView’s data - read further.
The Chart and the GridView will be populated with the same data source – List of Business Objects:
- public class MyDateObject
- {
- public double SampleValue { get; set; }
- public DateTime Date { get; set; }
- }
-
- public class MyDataContext
- {
- public const int min = 20;
- public const int max = 80;
- public ObservableCollection<MyDateObject> List { get; set; }
- Random r = new Random();
- DateTime baseDate = DateTime.Today;
-
- public MyDataContext()
- {
- this.CreateChartData();
- }
-
- private void CreateChartData()
- {
- List = new ObservableCollection<MyDateObject>();
- for (int i = 0; i < 15; i++)
- {
- List.Add(new MyDateObject() { SampleValue = r.Next(min, max), Date = baseDate.AddDays(i) });
- }
- }
- }
The binding is done in XAML. The Chart is bound via SeriesMappings which you can find described in our online documentation – DataBinding with Manual Series Mappings.
As for the GridView – several properties (relating grouping, selecting and sorting) are turned off for simplicity.
By handling the Filtered event of the GridView, you may set the GridView filter descriptors directly to the chart using the IFilterDescriptor interface:
- private void GridView1_Filtered(object sender, Telerik.Windows.Controls.GridView.GridViewFilteredEventArgs e)
- {
- this.RadChart1.FilterDescriptors.Clear();
-
- foreach (IFilterDescriptor descriptor in this.GridView1.FilterDescriptors)
- {
- this.RadChart1.FilterDescriptors.Add(descriptor);
- }
- }
As an alternative you may create a ComplexFilterDescriptor manually.
- CompositeFilterDescriptor chartFilterDescriptorCol = new CompositeFilterDescriptor();
- private void GridView1_Filtered(object sender, Telerik.Windows.Controls.GridView.GridViewFilteredEventArgs e)
- {
- if (e.Added.Count() > 0)
- {
- foreach (FilterDescriptor filter in e.Added)
- {
- FilterDescriptor gridFilterDescriptor = filter;
-
- chartFilterDescriptorCol.FilterDescriptors.Add(gridFilterDescriptor);
- chartFilterDescriptorCol.LogicalOperator = FilterCompositionLogicalOperator.Or;
- }
- }
- this.RadChart1.FilterDescriptors.Add(chartFilterDescriptorCol);
- }
The pictures below show the sample in action:
1. The Chart and the GridView on initial load:
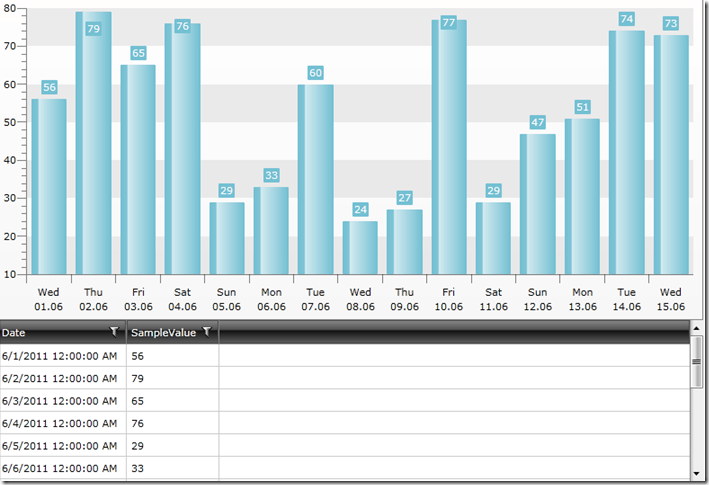
2. Filtering the SampleValue column:
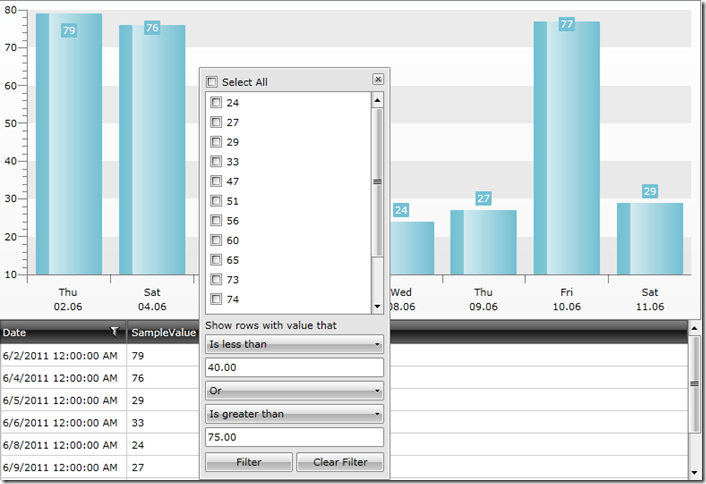
3. The result:
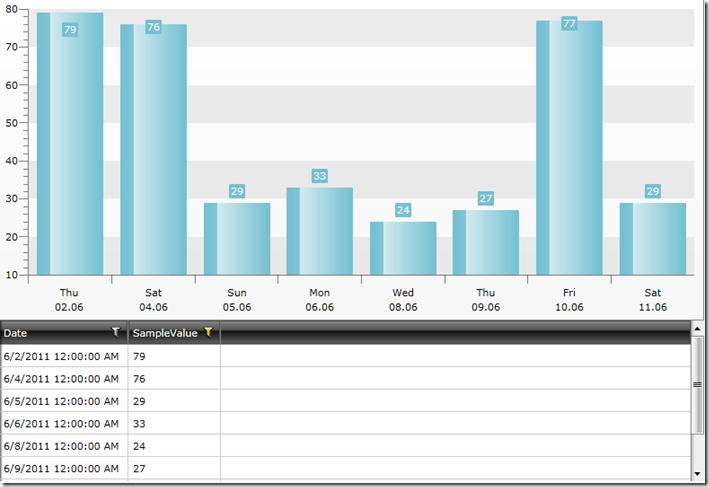
You may download the full source code from here!