Hey guys,
I am currently implementing a grouping in my Listview. The data is getting grouped by a Datetimevalue.
I can sort the Listview by using this Datetime but then I get the long Datetimevalue inside the header I tryed using stringformat and a valueconverter but without any effect.
Is there a way to modify the output in the groupingheader?
Best regards
Julian
5 Answers, 1 is accepted
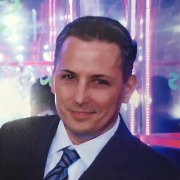
You can create a custom GroupHeaderTemplate with a Label to display the text.
<
telerikDataControls:RadListView.GroupHeaderTemplate
>
<
DataTemplate
>
<
Grid
>
<
Label
Text
=
"{Binding }"
/>
</
Grid
>
</
DataTemplate
>
</
telerikDataControls:RadListView.GroupHeaderTemplate
>
Now that you have you have access to the Label UI Element that renders the text, you can alter the bound text any way you see fit. One option you mentioned is to use a value converter to get the exact string format you want.
Demo
I've written a demo for you, find it attached. Here's a high level look at the relevant parts of the demo:
We convert the value to a string when it's bound to the header text, so you'll need to parse it back into a DateTime object, then return the newly formatted string.
Here's a converter using the "g" DateTime string format:
class
GroupHeaderDisplayFormatConverter : IValueConverter
{
public
object
Convert(
object
value, Type targetType,
object
parameter, CultureInfo culture)
{
// the bound header text is a string
var headerText = value
as
string
;
// get the valid DateTime object (dont use this in production, instead use a TryParse)
var parsedDateTime = DateTime.Parse(headerText);
// Return the formatted string using your preferred DataTime string format
return
parsedDateTime.ToString(
"g"
);
}
public
object
ConvertBack(
object
value, Type targetType,
object
parameter, CultureInfo culture)
{
throw
new
NotImplementedException();
}
}
Now, in your XAML, use the converter on Label's binding:
<
ContentPage.Resources
>
<
ResourceDictionary
>
<
converters:GroupHeaderDisplayFormatConverter
x:Key
=
"DisplayFormatConverter"
/>
</
ResourceDictionary
>
</
ContentPage.Resources
>
...
<
telerikDataControls:RadListView.GroupHeaderTemplate
>
<
DataTemplate
>
<
Grid
BackgroundColor
=
"#C1C1C1"
>
<
Label
Text
=
"{Binding Converter={StaticResource DisplayFormatConverter}}"
/>
</
Grid
>
</
DataTemplate
>
</
telerikDataControls:RadListView.GroupHeaderTemplate
>
Here's a screenshot of the result at run-time:
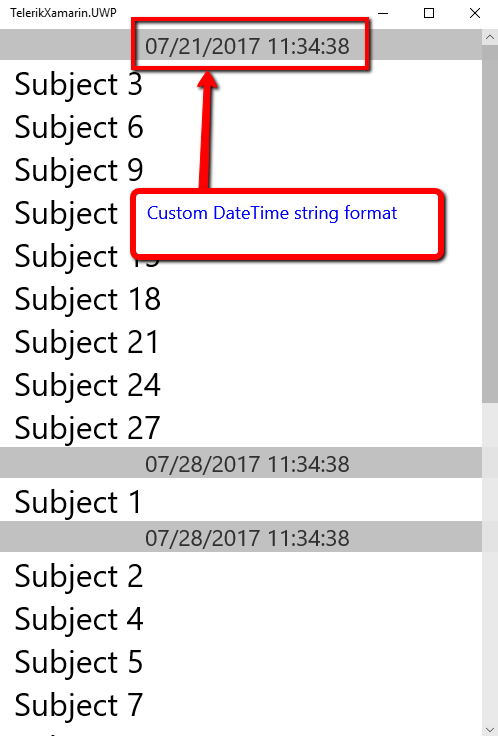
Regards,
Lance | Tech Support Engineer, Sr.
Progress Telerik

Thanks for your support!
This was exactly what I was looking for!
Best regards
Julian

You can find the documentation in RadListView Grouping (scroll down to the GroupHeaderTemplate section).
I'll talk to the development team to see if there's way to make this easier to find. Right now, we have it in the Grouping topic because that's where we would expect a developer to look for topics related to the grouping feature. However, it might be useful to have it also in the styling section.
Regards,
Lance | Technical Support Engineer, Principal
Progress Telerik

This is an old question, but I found it when I searched for a solution today.
With the current version of Telerik UI for Xamarin it's possible to use StringFormat instead of a custom converter.
<telerikDataControls:RadListView.GroupHeaderTemplate>
<DataTemplate>
<Label Text="{Binding Key, StringFormat='{0:D}'}" />
</DataTemplate>
</telerikDataControls:RadListView.GroupHeaderTemplate>
Hi Bernd,
Most definitely! since StringFormat is now an option available in Xamarin.Forms, it makes modifying the Key value for display easier and cleaner.