So I've got a Kendo Grid populated by a view model. All works fine when I am editing existing rows but when I try to add a new row I get a "Value cannot be NULL" error on my DropDownListFor control. The property for the selected id of the dropdownlist is a string and it is a non-nullable field in the database.
Here is my code:
ViewModel:
UserFacilities is my list for the dropdownlist and User_Facility_ID would be the selected item.
Controller:
This is the Get method for the grid which populates the ViewModel. I never get to the POST. It throws the error as soon as I click the "Add New Record" button on the grid.
EditorTemplate:
This is the DropDownListFor in my EditorTemplate being used on the grid.
I feel like I am missing something simple here...like somehow initializing the User_Facility_ID to an empty string or something so on a new record it doesn't have a null value which it is not allowed to have. I have tried doing this in the ViewModel but unless I was doing it wrong it didn't help.
Here is my code:
ViewModel:
public class ProposalTypeViewModel
{
public decimal ID { get; set; }
public string User_Facility_ID { get; set; }
public string Code { get; set; }
public string Description { get; set; }
public bool Active { get; set; }
public IEnumerable<
SelectListItem
> UserFacilities { get; set; }
}
Controller:
public ActionResult ProposalTypes()
{
var context = new PASSEntities();
User user = new User();
int userID = user.GetUserIDByBNLAccount(User.Identity.Name);
var model = (from a in context.Proposal_Types
join b in context.User_Facilities on a.User_Facility_ID equals b.ID
join c in context.Users_Roles on b.ID equals c.User_Facility_ID
join d in context.Users on c.User_ID equals d.ID
join e in context.Roles on c.Role_ID equals e.ID
where d.ID == userID && e.Name == "User_Facility_Admin"
select new ProposalTypeViewModel()
{
ID = a.ID,
User_Facility_ID = a.User_Facility_ID,
Code = a.Code,
Description = a.Description,
Active = a.Active,
UserFacilities = (from f in context.User_Facilities
join g in context.Users_Roles on f.ID equals g.User_Facility_ID
join h in context.Users on g.User_ID equals h.ID
join i in context.Roles on g.Role_ID equals i.ID
where h.ID == userID && i.Name == "User_Facility_Admin"
select new SelectListItem
{
Text = f.ID,
Value = f.ID
})
});
return View(model);
}
EditorTemplate:
@model PASSAdmin.ViewModels.UserFacilityAdmin.ProposalTypeViewModel
<
div
class
=
"editor-label"
>
@Html.Label("User Facility")
</
div
>
<
div
class
=
"editor-field"
>
@Html.DropDownListFor(model => model.User_Facility_ID, new SelectList(Model.UserFacilities, "Value", "Text"), "(Select One)")
@Html.ValidationMessageFor(model => model.User_Facility_ID)
</
div
>
I feel like I am missing something simple here...like somehow initializing the User_Facility_ID to an empty string or something so on a new record it doesn't have a null value which it is not allowed to have. I have tried doing this in the ViewModel but unless I was doing it wrong it didn't help.
14 Answers, 1 is accepted
0
Hello Stephen,
I would recommend you to check the following Code Library project:
Regards,
Alexander Popov
Telerik
I would recommend you to check the following Code Library project:
Regards,
Alexander Popov
Telerik
Join us on our journey to create the world's most complete HTML 5 UI Framework - download Kendo UI now!
0

Stephen
Top achievements
Rank 1
answered on 17 Oct 2013, 02:22 PM
I was testing that out using the Kendo DropDownListFor and what I realized is that its not my selected id being null that's a problem, it's that my selectlist itself is coming in null.
So the "Value cannot be NULL" error is actually being thrown on the Model.UserFacilities property:
@Html.DropDownListFor(model => model.User_Facility_ID, new SelectList(Model.UserFacilities, "Value", "Text"), "(Select One)")
So I guess it is the way it is being filled? If I debug, after the "Add New" is clicked on the grid and it goes back to my get method, I see that the UserFacilities property of my ViewModel is being filled properly, but somehow when it gets back to the grid it becomes null...??
0

Stephen
Top achievements
Rank 1
answered on 17 Oct 2013, 03:41 PM
If I fill my SelectList and store in ViewData instead of my ViewModel it works....but I don't want to do that. So how do I modify my ViewModel fill to make it work properly?
Here is what I have now in my get method for the grid:
I am doing that sort of nested select in the LINQ projection to fill the UserFacilities SelectList. Is there another way I can do it without using the ViewData/ViewBag? Again, this works on an edit, just not on an add.
Here is what I have now in my get method for the grid:
public ActionResult ProposalTypes()
{
var context = new PASSEntities();
User user = new User();
int userID = user.GetUserIDByBNLAccount(User.Identity.Name);
var model = (from a in context.Proposal_Types
join b in context.User_Facilities on a.User_Facility_ID equals b.ID
join c in context.Users_Roles on b.ID equals c.User_Facility_ID
join d in context.Users on c.User_ID equals d.ID
join e in context.Roles on c.Role_ID equals e.ID
where d.ID == userID && e.Name == "User_Facility_Admin"
select new ProposalTypeViewModel()
{
ID = a.ID,
User_Facility_ID = a.User_Facility_ID,
Code = a.Code,
Description = a.Description,
Active = a.Active,
UserFacilities = (from f in context.User_Facilities
join g in context.Users_Roles on f.ID equals g.User_Facility_ID
join h in context.Users on g.User_ID equals h.ID
join i in context.Roles on g.Role_ID equals i.ID
where h.ID == userID && i.Name == "User_Facility_Admin"
select new SelectListItem
{
Text = f.ID,
Value = f.ID
})
});
return View(model);
}
I am doing that sort of nested select in the LINQ projection to fill the UserFacilities SelectList. Is there another way I can do it without using the ViewData/ViewBag? Again, this works on an edit, just not on an add.
0
Hello Stephen,
Could you please provide a runnable project where the issue is reproduced? This would help us pinpoint the exact reason for this behavior.
Regards,
Alexander Popov
Telerik
Could you please provide a runnable project where the issue is reproduced? This would help us pinpoint the exact reason for this behavior.
Regards,
Alexander Popov
Telerik
Join us on our journey to create the world's most complete HTML 5 UI Framework - download Kendo UI now!
0

Stephen
Top achievements
Rank 1
answered on 22 Oct 2013, 04:19 PM
Ok I have created a runnable project to demonstrate this.
Once you run it there will be a link on the Index page called "Proposal Types". Click that and it takes you to the view with the grid. If you edit a record the User Facility dropdown works fine. If you try to add a new one you will get the error message.
I had to remove the "packages" and the "lib" folders from the solution folder as it was too large to upload. I'm not sure if those are necessary or if you have the references locally it will run anyway.
Once you run it there will be a link on the Index page called "Proposal Types". Click that and it takes you to the view with the grid. If you edit a record the User Facility dropdown works fine. If you try to add a new one you will get the error message.
I had to remove the "packages" and the "lib" folders from the solution folder as it was too large to upload. I'm not sure if those are necessary or if you have the references locally it will run anyway.
0
Accepted
Hi Stephen,
Thank you for the provided project. I examined it and noticed that User_Facility_ID DropDownList is populated from the Model. I am not sure what is the reason for using the Model instead of the ViewData to pass the UserFacilities list, however I would like to point out that when adding a new item all of its fields are null. This includes the UserFacilities field which is used for populating the DropDownList. I would recommend you to pass the data through the ViewData object, ensuring it will always be there, as shown in the example project I sent you in one of my previous replies.
Regards,
Alexander Popov
Telerik
Thank you for the provided project. I examined it and noticed that User_Facility_ID DropDownList is populated from the Model. I am not sure what is the reason for using the Model instead of the ViewData to pass the UserFacilities list, however I would like to point out that when adding a new item all of its fields are null. This includes the UserFacilities field which is used for populating the DropDownList. I would recommend you to pass the data through the ViewData object, ensuring it will always be there, as shown in the example project I sent you in one of my previous replies.
Regards,
Alexander Popov
Telerik
Join us on our journey to create the world's most complete HTML 5 UI Framework - download Kendo UI now!
0

Stephen
Top achievements
Rank 1
answered on 24 Oct 2013, 01:37 PM
I prefer not to use ViewData/ViewBag. Is there any other way that would work or is that my only option?
0
You could use Ajax Binding, for example:
Regards,
Alexander Popov
Telerik
The Controller:
public JsonResult GetUser_Facilities()
{
var
context =
new
TestEntities();
var
model = (from f
in
context.User_Facilities
select
new
SelectListItem
{
Text = f.ID,
Value = f.ID
});
return
Json(model, JsonRequestBehavior.AllowGet);
}
The EditorTemplate View:
@(Html.Kendo().DropDownListFor(m => m.User_Facility_ID)
//use the data-skip attribute to prevent adding the default value binding and add the data-bind attribute for the custom binder
.HtmlAttributes(
new
{ data_skip =
"true"
, data_bind =
"nullableValue: Value"
})
.OptionLabel(
"(Select One!)"
)
//.BindTo(new SelectList(Model.UserFacilities, "Value", "Text"))
.DataValueField(
"Value"
)
.DataTextField(
"Text"
)
.DataSource(source => {
source.Read(read =>
{
read.Action(
"GetUser_Facilities"
,
"UserFacilityAdmin"
);
});
})
)
Regards,
Alexander Popov
Telerik
Join us on our journey to create the world's most complete HTML 5 UI Framework - download Kendo UI now!
0

Stephen
Top achievements
Rank 1
answered on 28 Oct 2013, 02:28 PM
Ok thank you for the answers. So just so I understand going forward, when using the grid there is no way to fill the dropdownlist via my Model without ajax. The best option then is ViewData?
0
Yes, that is correct.
Regards,
Alexander Popov
Telerik
Regards,
Alexander Popov
Telerik
Join us on our journey to create the world's most complete HTML 5 UI Framework - download Kendo UI now!
0

Stephen
Top achievements
Rank 1
answered on 28 Oct 2013, 05:21 PM
Thanks
0

John
Top achievements
Rank 1
answered on 31 Dec 2018, 09:09 PM
Is this still the case as of 12/31/18 regarding "when using the grid there is no way to fill the dropdownlist via my Model without ajax."
0
Hi, John,
This is quite an old thread which focuses on ServerEditing.
https://demos.telerik.com/aspnet-mvc/Grid/ServerEditing
I tested a server editing scenario and had no issues passing the model collection around both to the Kendo UI DropDownList and to the DropDownList provided by the framework:
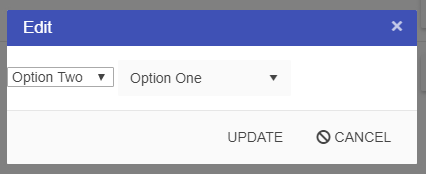
However, few projects these days use server rendering and editing, so I assume that the desired functionality is relevant to the Ajax bound grid.
https://demos.telerik.com/aspnet-mvc/grid/editing-popup
Using the ajax bound grid, the standard approach is to provide a data source or use the ViewData as it is done in the Foreign Key scenarios. If you wish to bind to the model items, there is one way to achieve it. During editing the Kendo UI MVVM framework is called upon to initialize the widgets on the form. So you may decorate the DropDownList with a data-bind attribute which will bind the available model collection to the data source:
https://docs.telerik.com/kendo-ui/framework/mvvm/bindings/source
Let me know in case you have further questions.
Regards,
Alex Hajigeorgieva
Progress Telerik
This is quite an old thread which focuses on ServerEditing.
https://demos.telerik.com/aspnet-mvc/Grid/ServerEditing
I tested a server editing scenario and had no issues passing the model collection around both to the Kendo UI DropDownList and to the DropDownList provided by the framework:
However, few projects these days use server rendering and editing, so I assume that the desired functionality is relevant to the Ajax bound grid.
https://demos.telerik.com/aspnet-mvc/grid/editing-popup
Using the ajax bound grid, the standard approach is to provide a data source or use the ViewData as it is done in the Foreign Key scenarios. If you wish to bind to the model items, there is one way to achieve it. During editing the Kendo UI MVVM framework is called upon to initialize the widgets on the form. So you may decorate the DropDownList with a data-bind attribute which will bind the available model collection to the data source:
https://docs.telerik.com/kendo-ui/framework/mvvm/bindings/source
@(Html.Kendo().DropDownListFor(model => model.Employees)
.DataTextField(
"EmployeeName"
)
.DataValueField(
"EmployeeID"
)
.HtmlAttributes(
new
{ data_bind =
"source:Employees"
}))
Let me know in case you have further questions.
Regards,
Alex Hajigeorgieva
Progress Telerik
Get quickly onboarded and successful with your Telerik and/or Kendo UI products with the Virtual Classroom free technical training, available to all active customers. Learn More.
0

John
Top achievements
Rank 1
answered on 03 Jan 2019, 12:36 PM
Thank you, Alex. That makes sense.