Data Grid
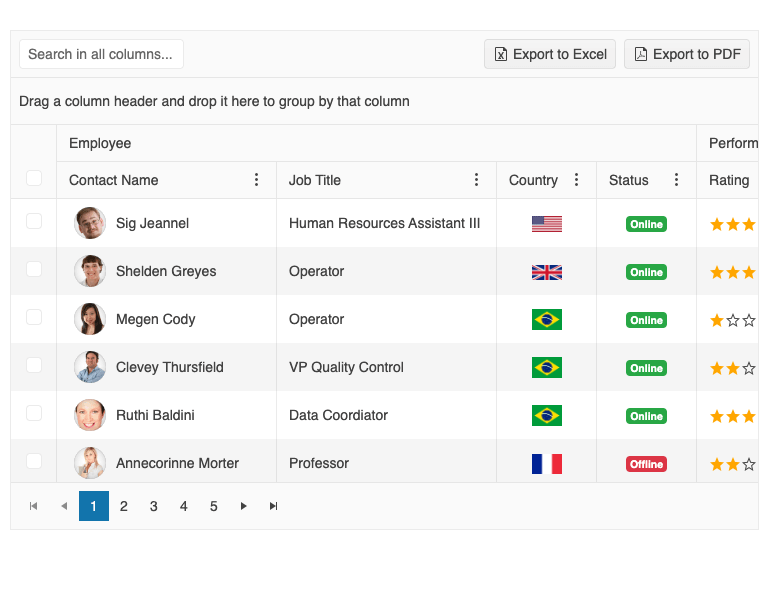
More developers rely on Kendo UI for Angular to deliver everything they need to build modern applications.
Greatness—it’s one thing to say you have it, but it means more when others recognize it. Kendo UI is proud to hold the following industry awards.
G2 Leaders
Summer Award
TrustRadius Most Loved Award
TrustRadius Best Feature Set Award
TrustRadius Best Usability Award
TrustRadius Best Customer Support Award
Kendo UI for Angular delivers components to meet any requirements including grids, data visualization, input and more. Each is carefully crafted to be the best of breed. Spend your time developing core functionality and leave UI to us. Check out some of the most popular below:
/* tslint:disable:max-line-length */
import { Component, OnInit, ViewChild } from '@angular/core';
import { DataBindingDirective } from '@progress/kendo-angular-grid';
import { process } from '@progress/kendo-data-query';
import { employees } from './employees';
import { images } from './images';
@Component({
selector: 'my-app',
template: `
<kendo-grid
[kendoGridBinding]="gridView"
kendoGridSelectBy="id"
[selectedKeys]="mySelection"
[pageSize]="20"
[pageable]="true"
[sortable]="true"
[groupable]="true"
[reorderable]="true"
[resizable]="true"
[height]="480"
[columnMenu]="{ filter: true }"
>
<ng-template kendoGridToolbarTemplate>
<input placeholder="Search in all columns..." kendoTextBox (input)="onFilter($event.target.value)"/>
<button kendoGridExcelCommand type="button" icon="file-excel" style="float:right;">Export to Excel</button>
<button kendoGridPDFCommand icon="file-pdf" style="float:right;">Export to PDF</button>
</ng-template>
<kendo-grid-checkbox-column
[width]="45"
[headerClass]="{'text-center': true}"
[class]="{'text-center': true}"
[resizable]="false"
[columnMenu]="false"
showSelectAll="true"
></kendo-grid-checkbox-column>
<kendo-grid-column-group title="Employee" [columnMenu]="false">
<kendo-grid-column field="full_name" title="Contact Name" [width]="220">
<ng-template kendoGridCellTemplate let-dataItem>
<div class="customer-photo" [ngStyle]="{'background-image' : photoURL(dataItem)}"></div>
<div class="customer-name">{{ dataItem.full_name }}</div>
</ng-template>
</kendo-grid-column>
<kendo-grid-column field="job_title" title="Job Title" [width]="220">
</kendo-grid-column>
<kendo-grid-column
field="country"
title="Country"
[width]="100"
[class]="{'text-center': true}"
[resizable]="false"
>
<ng-template kendoGridCellTemplate let-dataItem>
<img class="flag" [src]="flagURL(dataItem)" width="30">
</ng-template>
</kendo-grid-column>
<kendo-grid-column
field="is_online"
title="Status"
[width]="100"
[class]="{'text-center': true}"
[resizable]="false"
filter="boolean"
>
<ng-template kendoGridCellTemplate let-dataItem>
<span *ngIf="dataItem.is_online === true" class="badge badge-success">Online</span>
<span *ngIf="dataItem.is_online === false" class="badge badge-danger">Offline</span>
</ng-template>
</kendo-grid-column>
</kendo-grid-column-group>
<kendo-grid-column-group title="Performance" [columnMenu]="false">
<kendo-grid-column
field="rating"
title="Rating"
[width]="110"
[resizable]="false"
filter="numeric"
>
<ng-template kendoGridCellTemplate let-dataItem>
<kendo-rating
[value]="dataItem.rating"
[max]="5"
></kendo-rating>
</ng-template>
</kendo-grid-column>
<kendo-grid-column
field="target"
title="Engagement"
[width]="230"
[resizable]="false"
filter="numeric"
>
<ng-template kendoGridCellTemplate let-dataItem>
<kendo-sparkline type="bar"
[data]="dataItem.target"
[tooltip]="{visible: false}"
[transitions]="true"
[seriesDefaults]="{labels: {background: 'none', visible: true, format: '{0}%'}}"
>
<kendo-chart-area opacity="0" [width]="200"></kendo-chart-area>
<kendo-chart-value-axis>
<kendo-chart-value-axis-item [min]="0" [max]="130">
</kendo-chart-value-axis-item>
</kendo-chart-value-axis>
</kendo-sparkline>
</ng-template>
</kendo-grid-column>
<kendo-grid-column
field="budget"
title="Budget"
[width]="100"
filter="numeric"
>
<ng-template kendoGridCellTemplate let-dataItem>
<span [ngClass]="{'red text-bold': dataItem.budget < 0}">{{ dataItem.budget | currency }}</span>
</ng-template>
</kendo-grid-column>
</kendo-grid-column-group>
<kendo-grid-column-group title="Contacts" [columnMenu]="false">
<kendo-grid-column field="phone" title="Phone" [width]="130">
</kendo-grid-column>
<kendo-grid-column field="address" title="Address" [width]="200">
</kendo-grid-column>
</kendo-grid-column-group>
<kendo-grid-pdf fileName="Employees.pdf" [repeatHeaders]="true"></kendo-grid-pdf>
<kendo-grid-excel fileName="Employees.xlsx"></kendo-grid-excel>
</kendo-grid>
`,
styles: [`
.customer-photo {
display: inline-block;
width: 32px;
height: 32px;
border-radius: 50%;
background-size: 32px 35px;
background-position: center center;
vertical-align: middle;
line-height: 32px;
box-shadow: inset 0 0 1px #999, inset 0 0 10px rgba(0,0,0,.2);
margin-left: 5px;
}
.customer-name {
display: inline-block;
vertical-align: middle;
line-height: 32px;
padding-left: 10px;
}
.red {
color: #d9534f;
}
.text-bold {
font-weight: 600;
}
`]
})
export class AppComponent implements OnInit {
@ViewChild(DataBindingDirective) dataBinding: DataBindingDirective;
public gridData: any[] = employees;
public gridView: any[];
public mySelection: string[] = [];
public ngOnInit(): void {
this.gridView = this.gridData;
}
public onFilter(inputValue: string): void {
this.gridView = process(this.gridData, {
filter: {
logic: "or",
filters: [
{
field: 'full_name',
operator: 'contains',
value: inputValue
},
{
field: 'job_title',
operator: 'contains',
value: inputValue
},
{
field: 'budget',
operator: 'contains',
value: inputValue
},
{
field: 'phone',
operator: 'contains',
value: inputValue
},
{
field: 'address',
operator: 'contains',
value: inputValue
}
],
}
}).data;
this.dataBinding.skip = 0;
}
private photoURL(dataItem: any): string {
const code: string = dataItem.img_id + dataItem.gender;
const image: any = images;
return image[code];
}
private flagURL(dataItem: any): string {
const code: string = dataItem.country;
const image: any = images;
return image[code];
}
}
import{ Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<kendo-chart [categoryAxis]='{ categories: categories }'>
<kendo-chart-title text='Gross domestic product growth /GDP annual %/'></kendo-chart-title>
<kendo-chart-legend position='bottom' orientation='horizontal'></kendo-chart-legend>
<kendo-chart-tooltip format='{0}%'></kendo-chart-tooltip>
<kendo-chart-series>
<kendo-chart-series-item *ngFor='let item of series'
type='line' style='smooth' [data]='item.data' [name]='item.name'>
</kendo-chart-series-item>
</kendo-chart-series>
</kendo-chart>
`
})
export class AppComponent {
public series: any[] = [{
name: 'India',
data: [3.907, 7.943, 7.848, 9.284, 9.263, 9.801, 3.890, 8.238, 9.552, 6.855]
}, {
name: 'Russian Federation',
data: [4.743, 7.295, 7.175, 6.376, 8.153, 8.535, 5.247, -7.832, 4.3, 4.3]
}, {
name: 'Germany',
data: [0.010, -0.375, 1.161, 0.684, 3.7, 3.269, 1.083, -5.127, 3.690, 2.995]
}, {
name: 'World',
data: [1.988, 2.733, 3.994, 3.464, 4.001, 3.939, 1.333, -2.245, 4.339, 2.727]
}];
public categories: number[] = [2002, 2003, 2004, 2005, 2006, 2007, 2008, 2009, 2010, 2011];
}
import { Component } from '@angular/core';
import { SchedulerEvent } from '@progress/kendo-angular-scheduler';
import { sampleData, displayDate } from './events-utc';
@Component({
selector: 'my-app',
template: `
<kendo-scheduler [kendoSchedulerBinding]="events"
[selectedDate]="selectedDate"
scrollTime="08:00"
style="height: 600px;">
<kendo-scheduler-day-view>
</kendo-scheduler-day-view>
<kendo-scheduler-week-view>
</kendo-scheduler-week-view>
<kendo-scheduler-month-view>
</kendo-scheduler-month-view>
<kendo-scheduler-timeline-view>
</kendo-scheduler-timeline-view>
<kendo-scheduler-agenda-view>
</kendo-scheduler-agenda-view>
</kendo-scheduler>
`
})
export class AppComponent {
public selectedDate: Date = displayDate;
public events: SchedulerEvent[] = sampleData;
}
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
styles: ['.k-calendar { margin: 0 auto; }'],
template: `
<div class="row example-wrapper">
<div class="col-xs-12 col-md-6 example-col">
<p>Infinite Calendar</p>
<kendo-calendar [type]="'infinite'"></kendo-calendar>
</div>
<div class="col-xs-12 col-md-6 example-col">
<p>Classic Calendar</p>
<kendo-calendar [type]="'classic'"></kendo-calendar>
</div>
</div>
`
})
export class AppComponent { }
import { Component } from '@angular/core';
import { Employee, employees } from './employees';
@Component({
selector: 'my-app',
template: `
<div class="example-wrapper" style="min-height: 400px;">
<div class="col-xs-12 col-sm-6 example-col">
<p>AutoComplete</p>
<kendo-autocomplete [data]="listItems" [placeholder]="'Your favorite sport'">
</kendo-autocomplete>
</div>
<div class="col-xs-12 col-sm-6 example-col">
<p>ComboBox</p>
<kendo-combobox [data]="listItems" [value]="'Basketball'">
</kendo-combobox>
</div>
<div class="col-xs-12 col-sm-6 example-col">
<p>DropDownList</p>
<kendo-dropdownlist [data]="listItems" [value]="'Basketball'">
</kendo-dropdownlist>
</div>
<div class="col-xs-12 col-sm-6 example-col">
<p>DropDownTree</p>
<kendo-dropdowntree
kendoDropDownTreeExpandable
[kendoDropDownTreeHierarchyBinding]="treeItems"
textField="text"
valueField="id"
childrenField="items"
[value]="complexValue"
[popupSettings]="{
width: '230px'
}"
[expandedKeys]="['1']"
></kendo-dropdowntree>
</div>
<div class="col-xs-12 col-sm-6 example-col">
<p>MultiColumnComboBox</p>
<kendo-multicolumncombobox
[data]="gridData"
[textField]="'name'"
[valueField]="'id'"
[placeholder]="'Select an employee'"
>
<kendo-combobox-column
[field]="'name'"
[title]="'Name'"
[width]="200"
>
</kendo-combobox-column>
<kendo-combobox-column
[field]="'title'"
[title]="'Title'"
[width]="200"
>
</kendo-combobox-column>
<kendo-combobox-column
[field]="'phone'"
[title]="'Phone'"
[width]="200"
>
</kendo-combobox-column>
</kendo-multicolumncombobox>
</div>
<div class="col-xs-12 col-sm-6 example-col">
<p>MultiSelect</p>
<kendo-multiselect [data]="listItems" [value]="value" [placeholder]="'Your favorite sports'"></kendo-multiselect>
</div>
</div>
`
})
export class AppComponent {
public listItems: Array<string> = [
'Baseball', 'Basketball', 'Cricket', 'Field Hockey',
'Football', 'Table Tennis', 'Tennis', 'Volleyball'
];
public gridData: Employee[] = employees;
public treeItems: any[] = [
{
text: 'Furniture', id: 1, items: [
{ text: 'Tables & Chairs', id: 2 },
{ text: 'Sofas', id: 3 },
{ text: 'Occasional Furniture', id: 4 }
]
},
{
text: 'Decor', id: 5, items: [
{ text: 'Bed Linen', id: 6 },
{ text: 'Carpets', id: 7 }
]
}
];
public value = ['Basketball', 'Cricket'];
public complexValue = { text: 'Decor', id: 5 };
}
We know you have many choices when it comes to Angular UI. That’s why we go the extra mile ensure that your experience is enjoyable, successful, and problem-free.
Themes and Figma UI Kits
+ UI Kit for Figma
+ UI Kit for Figma
+ UI Kit for Figma
This is just a sampling of the more popular components. Start a trial to see them all.
The Pager is standalone UI component that gives you capability to split content and visualize it beautifully into pages. The Pager has multiple configuration options with which you can define the total number of items, number of items per page, number of visible page buttons, and support for loading data on demand for each page. You can integrate paging with other Blazor components easily – just like we do with our Grid and ListView!
A version of the dropdown that suggests items as the user types. Great for ensuing items are as finable as they can be.
The ComboBox shows a list of options the user can select from. They can easily filter that list to find data faster, or even input their own text. You can customize the appearance of the items and the selection through templates, implement your own custom filtering and load data on demand, react to events, validate the user input and benefit from built-in support for web accessibility standards and keyboard navigation.
The Calendar is a component that shows the days in a month, months in a year or even the years in a decade. It provides various calendar view options—month, year, and decade view—to help you quickly navigate to the desired date. The Calendar supports minimum dates, maximum dates, and disabled dates to restrict specific date selection. You can browse through the time, select dates and see all of that in your own culture settings and language.
Sign up for a trial and try the Data Grid and the rest of the Kendo UI for Angular library for yourself. Trials are free, fully supported, and only take a few minutes to start. You trial includes:
Since you are on a mobile device you cannot download your trial. Please enter your email and we will send you a link to download the trial.