In my first blog post I will GridView extension that many people requested similar to ASP.NET UI experience – executing CRUD operations using buttons to execute. Bellow I will show one option to achieve this easily using the implementation of the Command pattern and attached behaviors approach available in Silverlight and WPF.
Short overview of the command pattern
Command pattern encapsulates a request as an object and gives it a known public interface. Using this pattern ensures that every object receives its own commands and provides a decoupling between sender and receiver. A sender is an object that invokes an operation (usually called Invoker), and a receiver is an object that receives the request and acts on it. The idea can be easily represented by the following diagram:
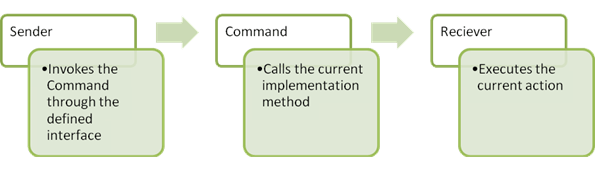
Using commands to create Custom Command Row for RadGridView
Using telerik RadControls offers you common implementation for WPF and Silvelight Command pattern. Using CommandManager implementation RadGridView offers you ability to use all of its commands and create additional row in the header providing easy access to operations such as edit, delete,insert, etc. We will use the following commands*:
- Delete – deletes current selected item
- BeginInsert – insert new row enabling to add new item to the grid
- BeginEdit – start editing current selected item
- CancelRowEdit – cancel edit or insert action
- CommitEdit – save any changed data of the current item
What we need to provide is to extend the behavior of the grid by adding our new row. We can do this with the well known approach of the behaviors. Here is the structure of our GridViewCommandRow class:
public class GridViewCommandRow
{
private ContentControl CommandRow { get; set; }
private RadGridView radGridView;
public GridViewCommandRow(RadGridView grid)
{
this.RadGridView = grid;
this.CommandRow = new ContentControl();
}
public static readonly DependencyProperty IsEnabledProperty =
DependencyProperty.RegisterAttached("IsEnabled",
typeof(bool),
typeof(GridViewCommandRow),
new PropertyMetadata(false, OnIsEnabledChanged));
private static void OnIsEnabledChanged(DependencyObject sender, DependencyPropertyChangedEventArgs e)
{
RadGridView radGridView = sender as RadGridView;
if (radGridView != null)
{
if ((bool)e.NewValue)
{
// Create new GridViewCommandRow and attach RadGridView events event.
GridViewCommandRow commandRow = new GridViewCommandRow(radGridView);
commandRow.Attach();
}
}
}
private void Attach()
{
this.RadGridView.DataLoaded += new EventHandler<EventArgs>(RadGridView_DataLoaded);
}
void RadGridView_DataLoaded(object sender, EventArgs e)
{ // Insert our row in the structure of the grid
}
With this code we have created mechanism to add our row inside the grid. The next step is to attach our Row to the grid. This is achieved using the this XAML:
<telerik:RadGridView local:GridViewCommandRow.IsEnabled="true"/>
Then we define two properties for the templates of the row for edit and view actions. That way we will be able to change and define different templates for the row making it completely customizable. Making it we need to keep in mind that we need to define the commands which will be called when pressing on the buttons. Since the standard Silverlight buttons does not support Commands we will use RadButtons. One of them looks like:
<telerik:RadButton Command="grid:RadGridViewCommands.BeginInsert"
Width="50" Height="20" Margin="5" telerik:StyleManager.Theme="{StaticResource Theme}" Content="Insert">
And finally assembling all parts together we get the following result:
And the end some additional ideas for Silverlight 4 fans– since Silverlight 4 Domain Data Source supports save data command you can use similar approach to save your modified data.
You can download the source from here:
download